Contents
Outline
In this blog post, I will introduce how to use flutter_colorpicker
to implement a feature that helps users to choose the color easily in Flutter.
- flutter_colorpicker: https://pub.dev/packages/flutter_colorpicker
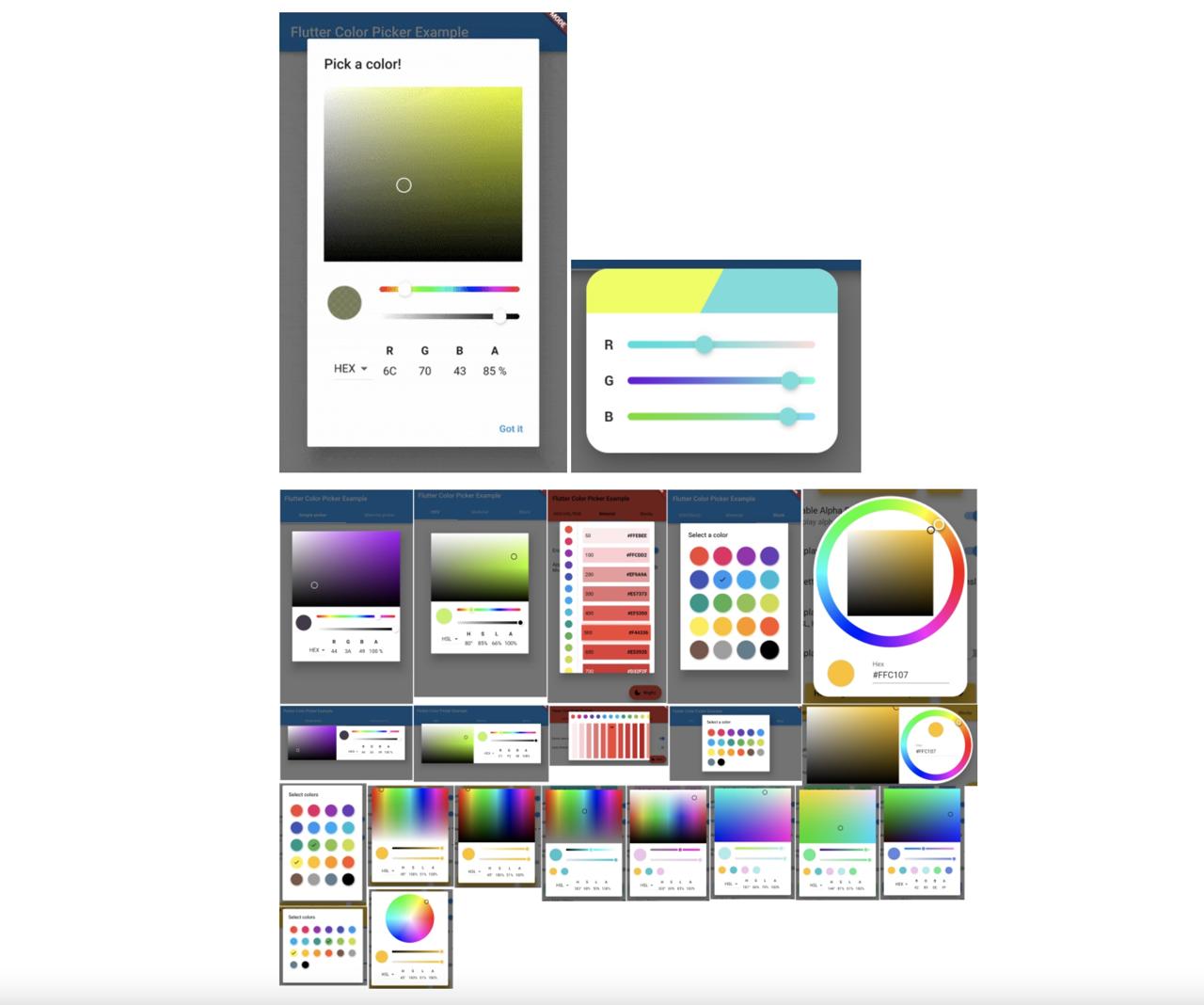
You can see the full source code of the blog post in the following link.
Official example
flutter_colorpicker
provides various features and examples to check the features. You can see the official example on the following link.
- Official example: https://github.com/mchome/flutter_colorpicker/tree/master/example
If you execute the example, you can see the following result.
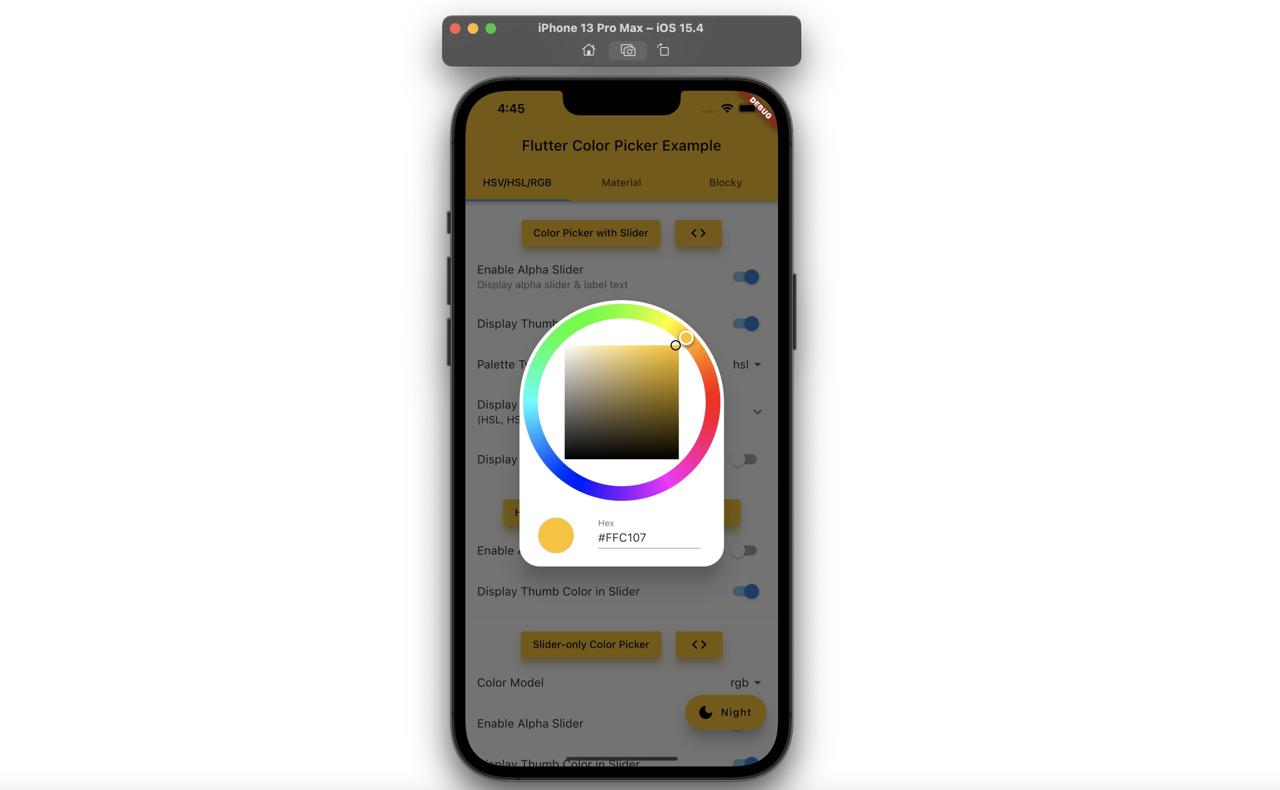
Also, flutter_colorpicker
supports the web platform, so you can see the example on the web like the below.
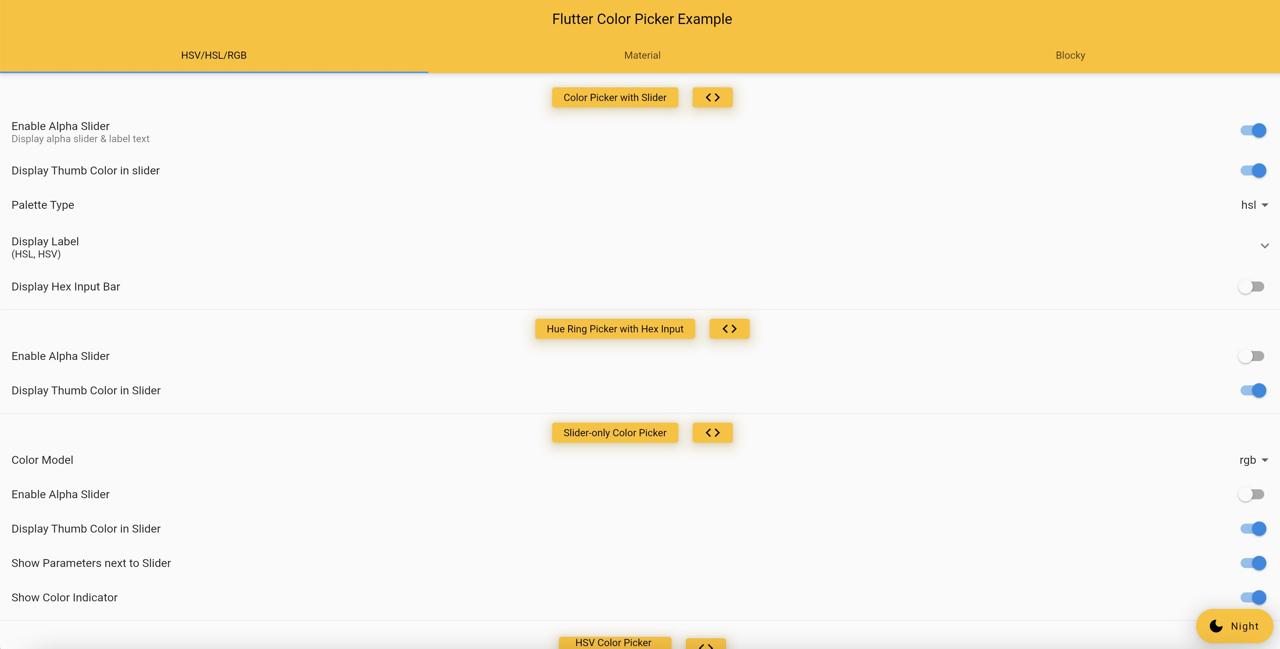
About the web example, you can see the following link.
- Web official example: https://mchome.github.io/flutter_colorpicker/
Simple example
flutter_colorpicker
provides various features, so the official example is a little bit complicated. So, we will make a simple example together in here to learn how to use flutter_colorpicker
.
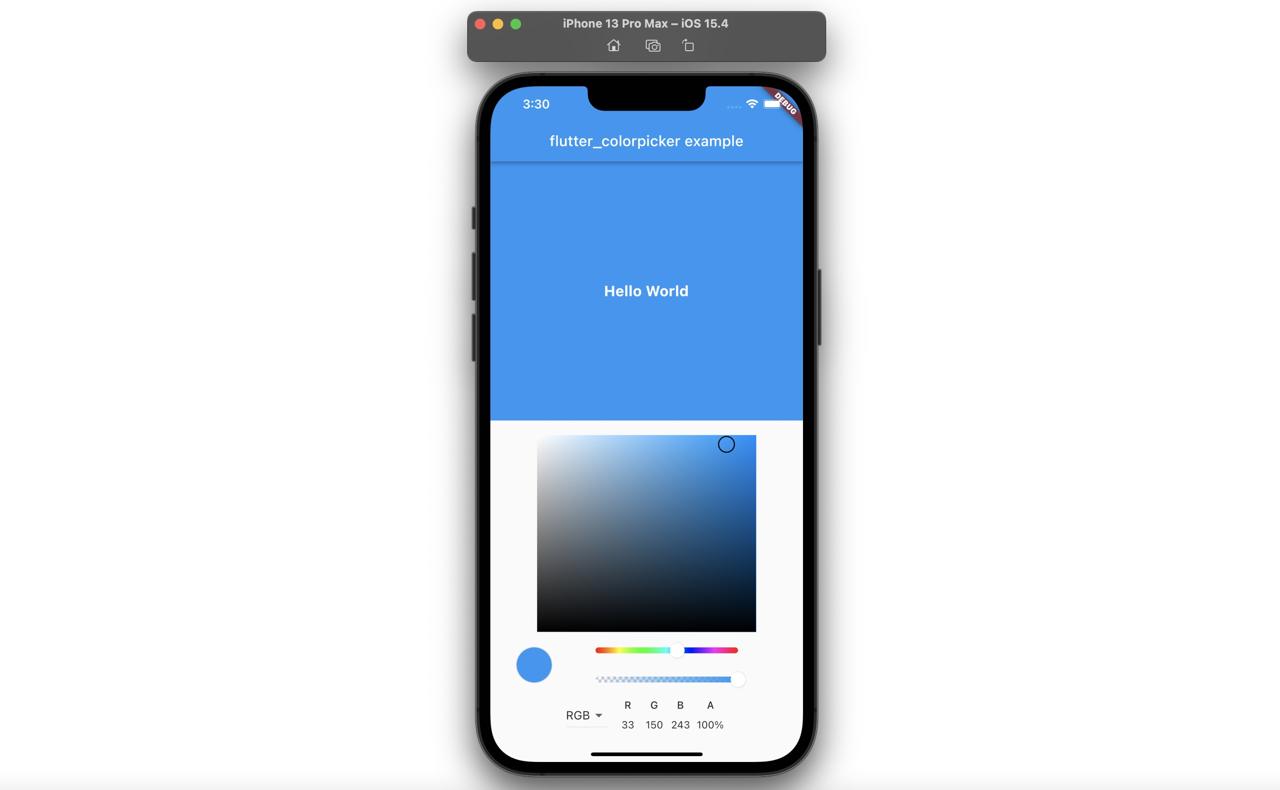
This example has flutter_colorpicker
on the bottom, and if the user changes the color, the Hello world
background color will be changed.
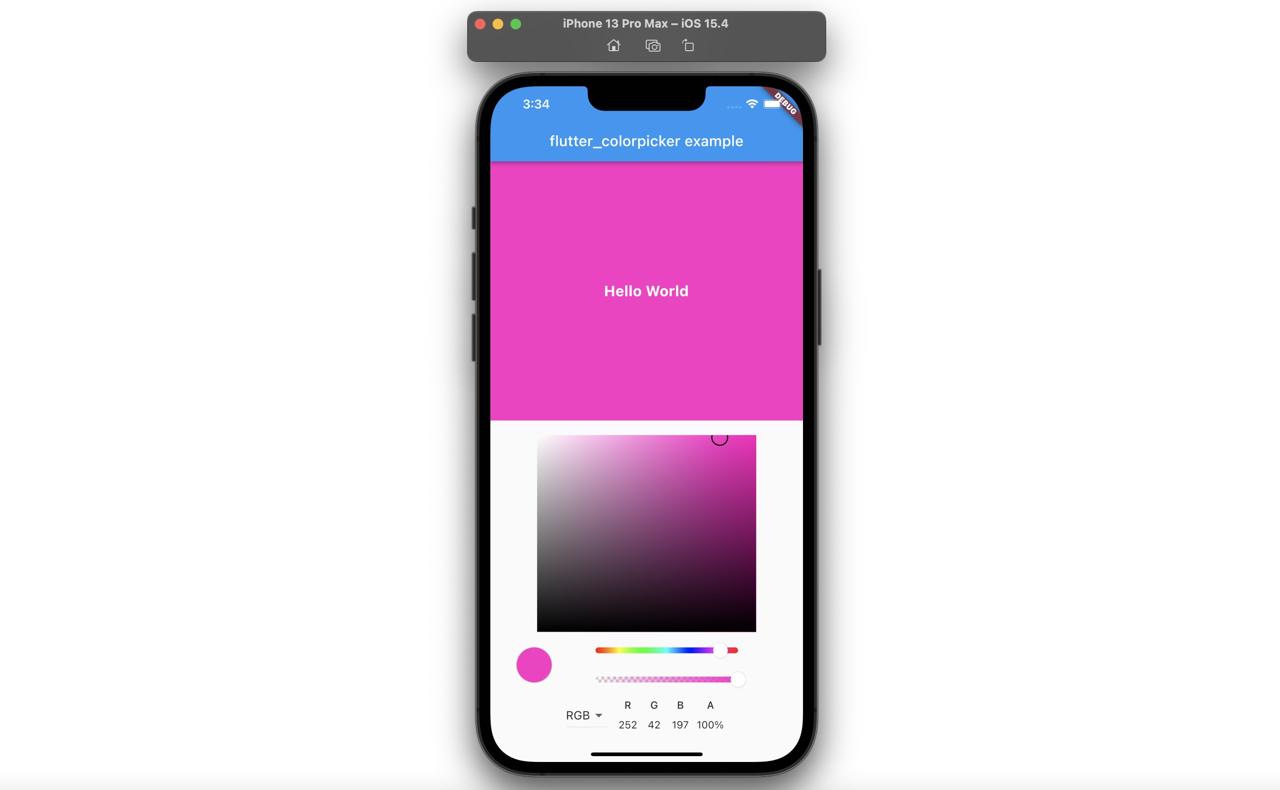
Create Flutter project
In Flutter, to implement the feature that users can change the color easily with flutter_colorpicker
, we’ll create a new Flutter project. Execute the following command to create a new Flutter project.
flutter create flutter_colorpicker_example
Install flutter_colorpicker
To use flutter_colorpicker
, execute the following command to install the flutter_colorpicker
package.
# cd flutter_colorpicker_example
flutter pub add flutter_colorpicker
Implement example
Now, let’s implement an example with flutter_colorpicker
. To implement the example, open the ./lib/main.dart
file and modify it like the below.
...
class _MyHomePageState extends State<MyHomePage> {
Color _color = Colors.blue;
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(widget.title),
),
body: Center(
child: Column(
children: [
Expanded(
flex: 1,
child: Container(
width: double.infinity,
color: _color,
child: const Center(
child: Text(
"Hello World",
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
color: Colors.white,
),
),
),
),
),
Padding(
padding: const EdgeInsets.all(20.0),
child: ColorPicker(
pickerColor: _color,
onColorChanged: (Color color) {
setState(() {
_color = color;
});
},
pickerAreaHeightPercent: 0.9,
enableAlpha: true,
paletteType: PaletteType.hsvWithHue,
),
),
],
),
),
);
}
}
In this example, the color that the user chooses with flutter_colorpicker
will be stored in the State
, and it will be used to change the color in the screen.
class _MyHomePageState extends State<MyHomePage> {
Color _color = Colors.blue;
...
}
The screen has the Column
widget. And it has a widget on the top that shows the color that the user chooses with flutter_colorpicker
, and flutter_colorpicker
is shown on the bottom.
...
@override
Widget build(BuildContext context) {
return Scaffold(
...,
body: Center(
child: Column(
children: [
Expanded(
flex: 1,
child: Container(
width: double.infinity,
color: _color,
child: const Center(
...
),
),
),
),
Padding(
padding: const EdgeInsets.all(20.0),
child: ColorPicker(
...
),
),
],
),
),
);
}
When the user chooses the color in the flutter_colorpicker
widget, the color will be stored in the State
, and the stored color will be used the background color of the Container
widget. And the white color text(Hello world
) will be shown on it.
...
child: Container(
width: double.infinity,
color: _color,
child: const Center(
child: Text(
"Hello World",
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
color: Colors.white,
),
),
),
),
...
To show flutter_colorpicker
, you need to use the ColorPicker
widget.
...
child: ColorPicker(
pickerColor: _color,
onColorChanged: (Color color) {
setState(() {
_color = color;
});
},
pickerAreaHeightPercent: 0.9,
enableAlpha: true,
paletteType: PaletteType.hsvWithHue,
),
...
When you use the ColorPicker
widget, you can set the default color to the pickerColor
parameter. And, when the color is changed in the ColorPicker
widget, the onColorChanged
function is called and the chosen color is passed to the parameter.
In this example, the chosen color is stored in the State
by the setState
function to change the background color of the widget.
Check
When you execute the example, you can see the blue background color and the Hello world
text is shown on it.
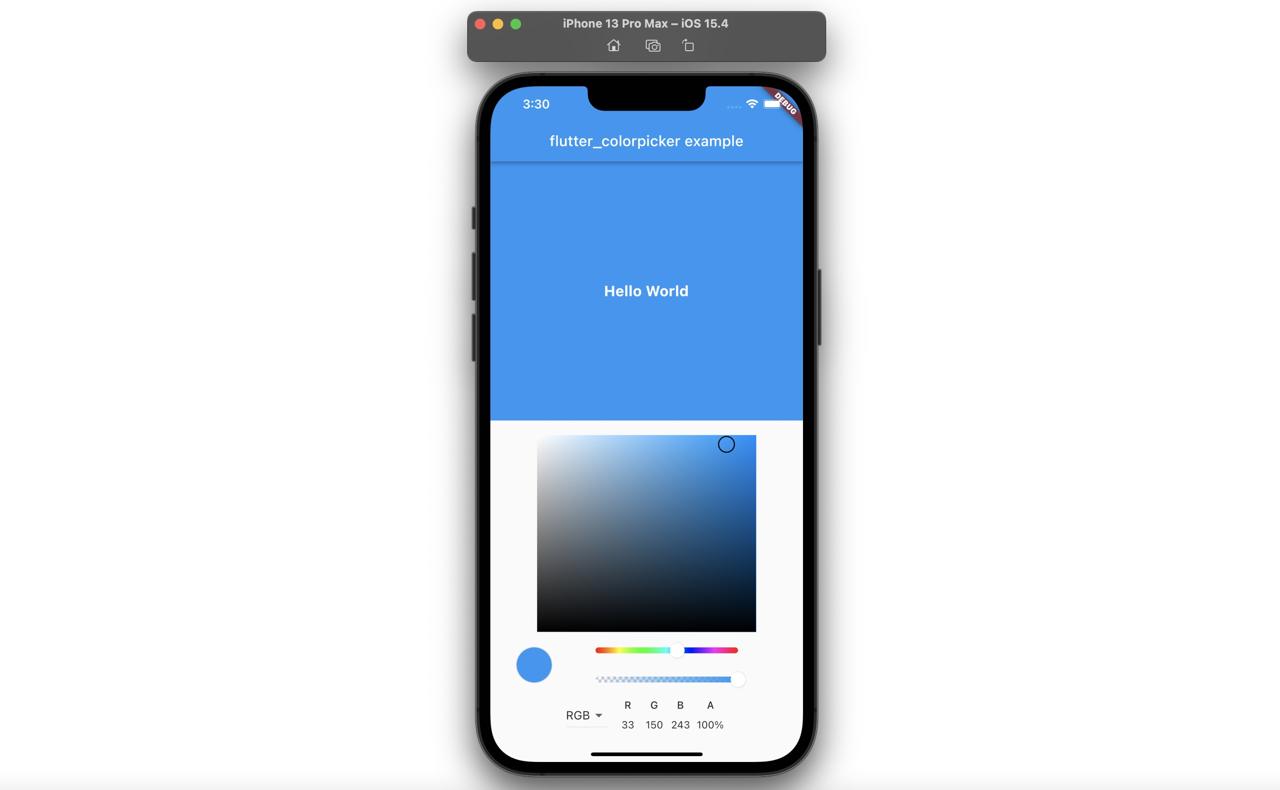
Next, when you change the color by the ColorPicker
widget, you can see the background color is changed well like the below.
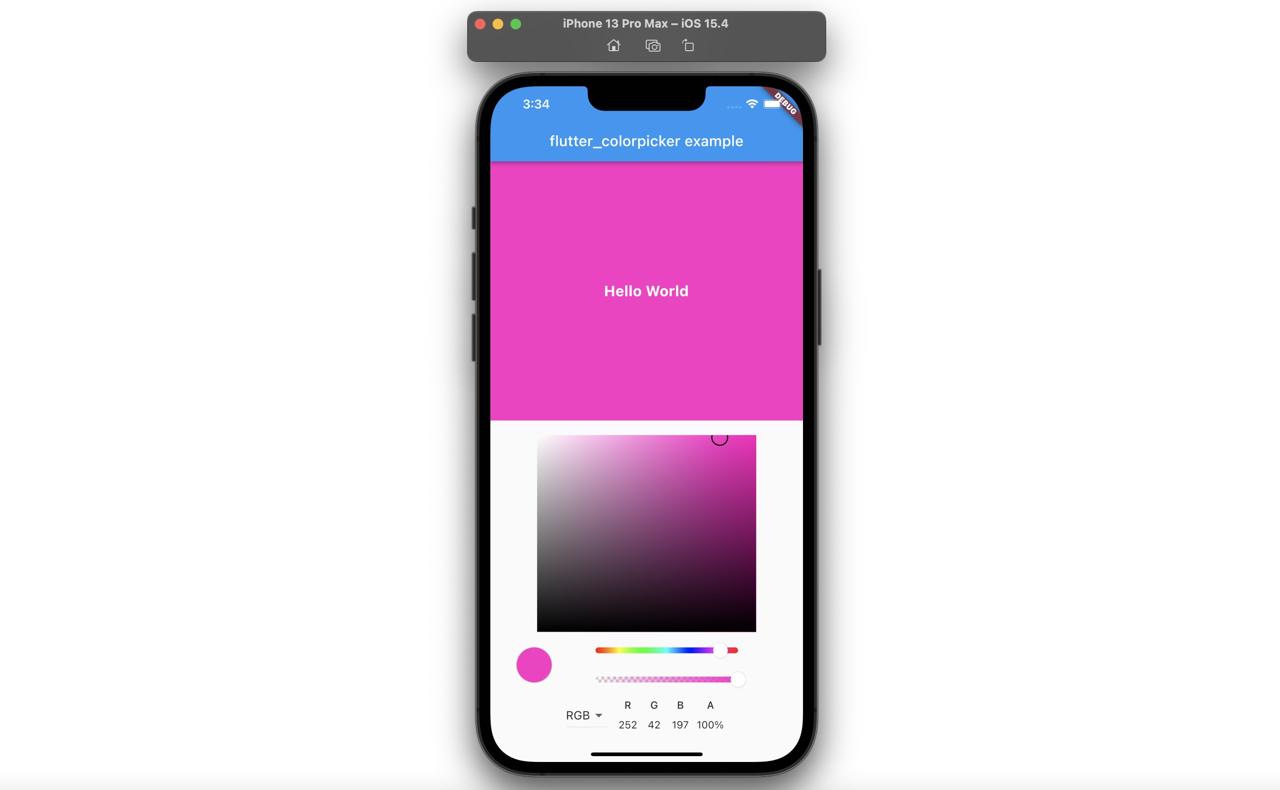
Completed
Done! we’ve seen how to use flutter_colorpicker
in Flutter
to implement the feature that helps the users change the color easily. flutter_colorpicker
provides various features. So, to implement the feature more easily, use my simple example to show it first, and try to find the features which you want to implement on the official example and add it to the simple example.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.