Contents
Outline
In web development, cache plays an important role. By using cache, you can improve the performance of web services and enhance user experience. In this blog post, I will introduce what cache is in web development and how to apply cache when providing web services with static files using AWS S3.
Background of Web Cache
To get data from the server, clients (PCs, smartphones) use the Internet.
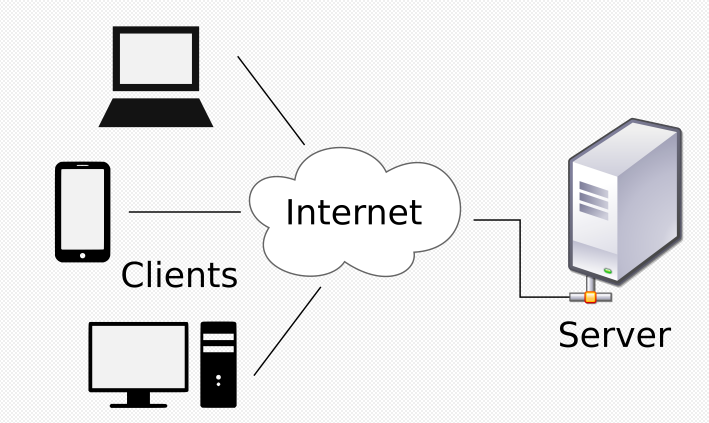
The Internet that connects clients and servers is physically connected, and the global Internet is connected by submarine cables.
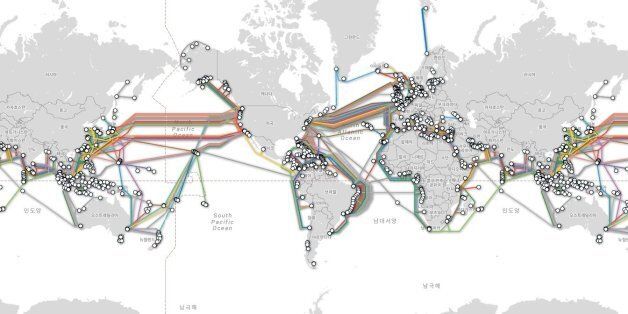
Clients and servers are physically connected, so it takes time to send and receive data as the distance increases.
Web Cache
Web cache is a technology that reduces data transmission delays by copying and placing content, that changes less frequently (static content: HTML, CSS, JS, Image, Video, etc.), from a server that is physically far away to a server that is physically close.
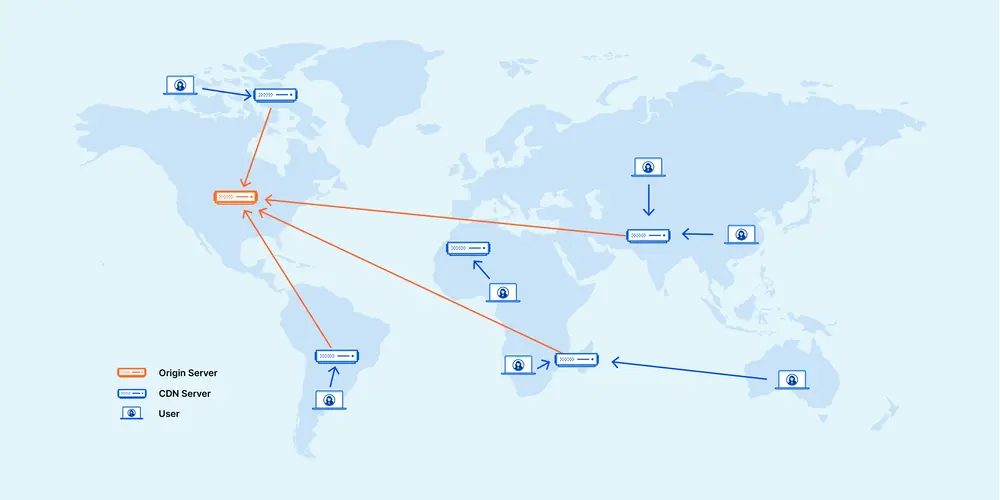
In web development, there are three main caches.
- CDN(Contents Delivery Network)
- Browser cache
- Database cache
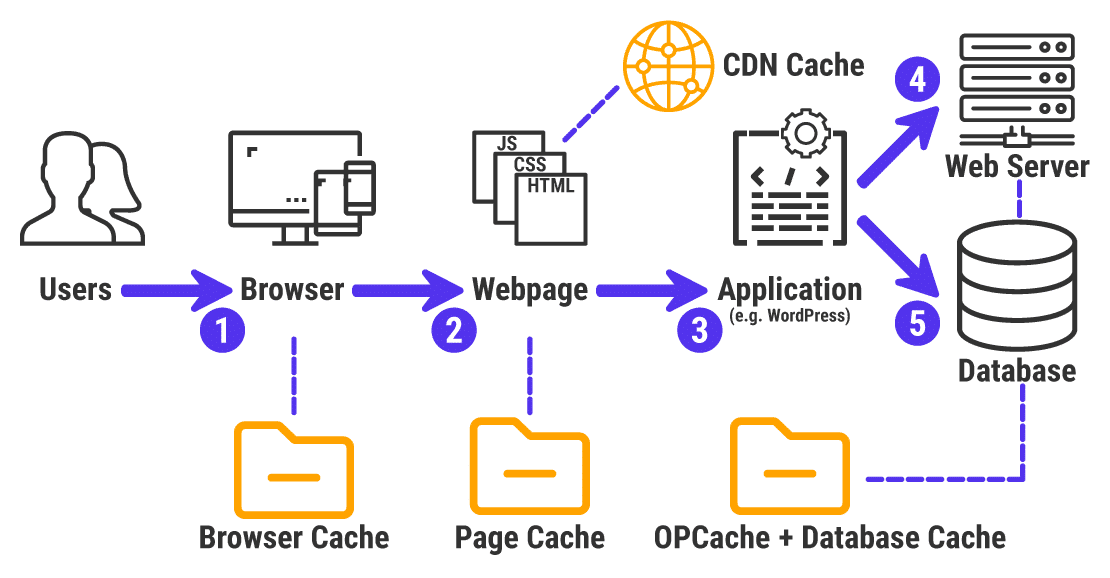
In this blog post, I will introduce CDN and browser cache.
CDN(Contents Delivery Network)
CDN is a service that provides content by copying less frequently changing content to servers in various parts of the world.
This reduces the distance between the client and the server, reducing the data transmission and reception delay.
For example, the world-famous CDN service Cloudflare
has servers around the world as follows.
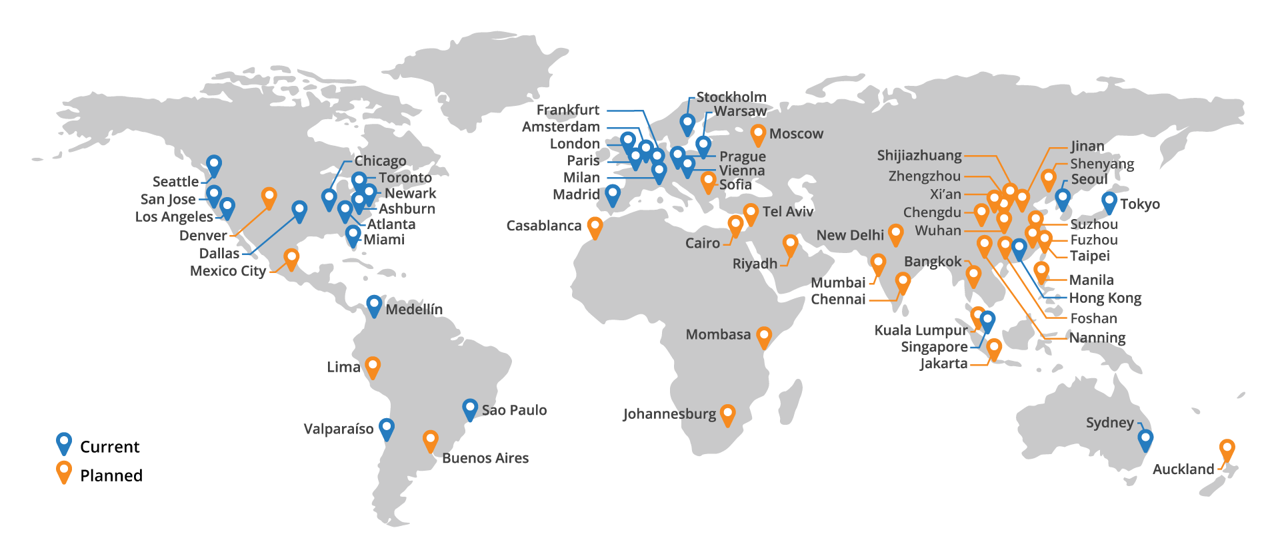
If you use Cloudflare
, you can copy static files to the server provided by Cloudflare
and provide content from a server close to the client.
Browser cache
On the client’s side, the nearest server is the client itself. The browser provides a browser cache that stores some content in client storage from a site once accessed and allows this stored content to be used when re-accessed.
The Chrome browser caches content in the following locations.
- Windows:
C:\Users\<User Name>\AppData\Local\Google\Chrome\User Data\Default\Cache
- macOS:
/Users/<User Name>/Library/Caches/Google/Chrome/Default/Cache
How web cache works
Let’s take a look at how web cache works.
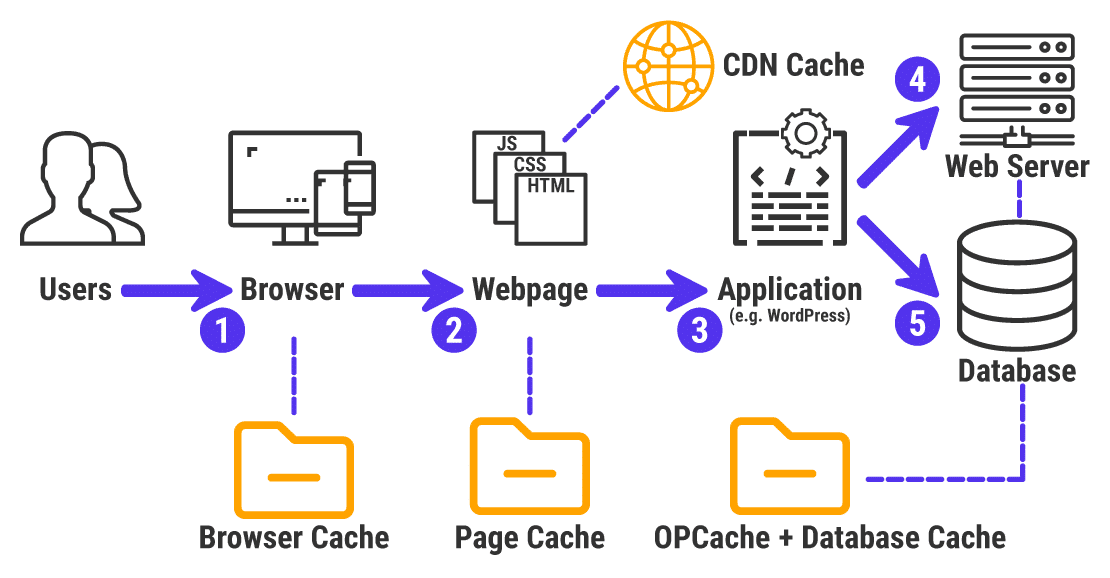
- The user accesses the service from the browser.
- The browser checks if there is anything cached in the browser and displays it if there is a cache.
- If there is no cache in the browser, check if there is a cache in the CDN. If there is a cache in the CDN, use it.
- If there is no cache in the CDN, get the content from the actual server.
- At this time, the CDN checks if it needs to cache and caches it if it can.
- If the browser can cache, it caches the content.
- From the next time the user accesses the same content, the browser and CDN use the cached content.
Web cache configuration
How do browsers and CDNs set caches? Browsers and CDNs determine whether to cache based on Expires
, Cache-Control
, Etag
, Last-Modified
, etc. in the HTTP response headers.
You can set the cache in the HTTP response header as follows.
- Nginx configuration
server {
listen 80;
server_name example.com;
location / {
# Set Cache-Control
add_header Cache-Control "max-age=3600, public";
# Set Expires
expires 1h;
}
}
- Web server configuration(NodeJS’s Express example)
const express = require('express');
const app = express();
app.use((req, res, next) => {
// Set Cache-Control
res.setHeader('Cache-Control', 'max-age=3600, public');
// Set Expires
const maxAgeInSeconds = 3600;
const date = new Date();
date.setSeconds(date.getSeconds() + seconds);
const expiryDate = date.toUTCString()
res.setHeader('Expires', expiryDate);
next();
});
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
If you set the HTTP response header like this, the CDN and the browser will set the cache.
AWS S3 and CloudFront
You can provide a static website using AWS’s S3 and CloudFront.
- S3(Simple Storage Service): A storage service for storing static files
- CloudFront: CDN service
AWS S3 is a storage service, but it also provides server functions that can serve web services with static files.
Typically, static web pages developed with HTML, CSS, JavaScript or web applications developed with frontend frameworks such as React, Vue, Angular, etc., are uploaded to S3 and served through CloudFront.
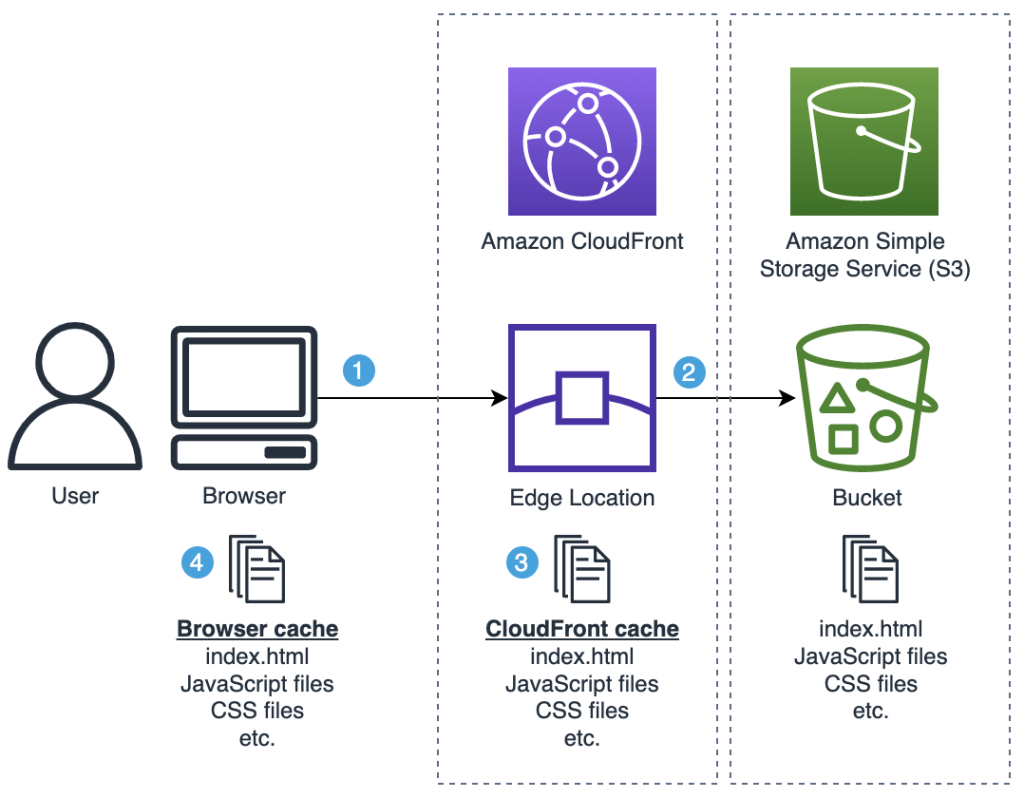
At this time, you can configure the uploaded files settings to set browser cache and CDN(CloudFront) cache.
AWS S3 Cache Settings
The cache is set by setting Expires
or Cache-Control
in the HTTP response header, so basically, it cannot be set on the frontend side.
In the case of CloudFront + S3
, since there is no server, you cannot add server code to set HTTP headers.
However, S3
has server functions, so you can configure the cache by changing the S3 settings.
When uploading files to S3 using the CLI tool provided by AWS, you can use the following command. In this case, you can set the cache using the --cache-control
option.
aws s3 cp <Target directory> s3://<S3 Bucket name> --recursive --exclude "assets/*" --cache-control 'public,max-age=60,stale-while-revalidate=2592000’
Target directory
: Local folder path where the build result to be uploaded to S3 is locatedS3 Bucket name
: S3’s Bucket name where the file will be uploaded--recursive
option:aws s3 cp
is a command that copies a single file by default, so use the--recursive
option to upload all files in the specified directory--exclude "assets/*"
: Set the upload exclusion target--cache-control 'public,max-age=60,stale-while-revalidate=2592000'
: Cache settingsmax-age=60
: Cache for 60 secondsstale-while-revalidate
: If the cache is expired bymax-age
, while revalidating the content in the background, provide the expired cache for up to 30 days
GitHub Actions
The following is the code to upload files to S3 using GitHub Actions
.
- name: Configure AWS credentials
uses: aws-actions/configure-aws-credentials@v4
with:
role-to-assume: $
aws-region: ap-northeast-1
- name: Upload file to S3
run: |
aws s3 rm s3://$ --recursive
aws s3 cp apps/dist s3://$ --recursive --exclude "assets/*" --cache-control 'public,max-age=60,stale-while-revalidate=2592000'
aws s3 sync apps/dist/assets s3://$/assets --cache-control 'public,max-age=60,immutable'
After deleting all files using aws s3 rm
, upload files using aws s3 cp
. Finally, upload the assets
folder using aws s3 sync
.
stale-while-revalidate for no downtime deployment
AWS introduced the usage of stale-while-revalidate
in the following blog post.
You can update the service version without interruption by using stale-while-revalidate
.
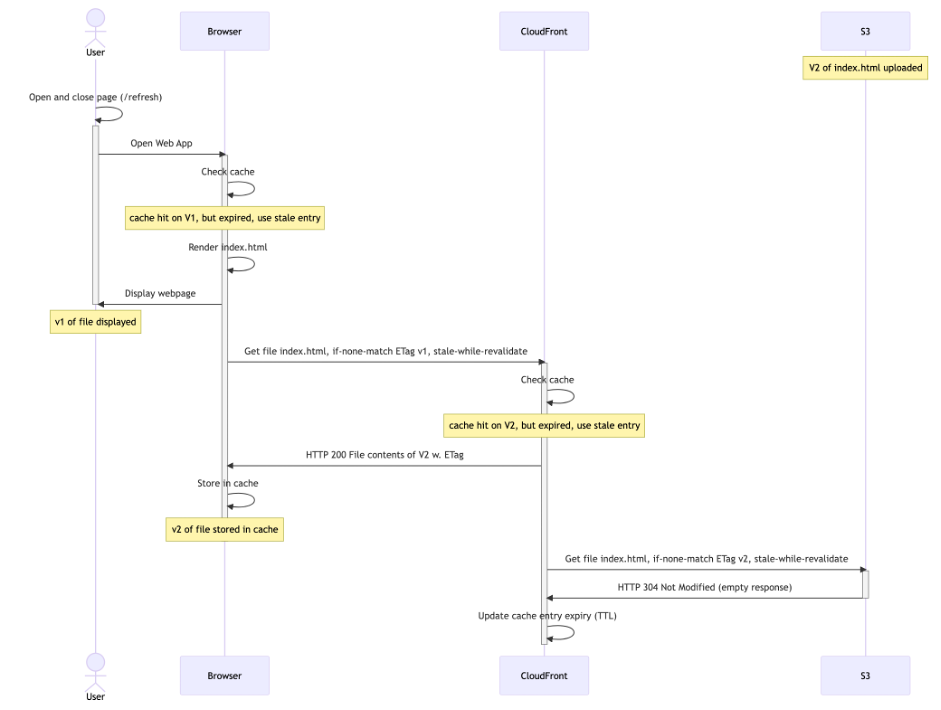
- The user runs the service in the browser.
- If the user is already using the service in V1 and has a cache of V1 with stale-while-revalidate in the browser, it will be used first.
- The browser requests content that has expired(max-age) from CloudFront(CDN) in the background.
- If there is a cache updated to V2 in CloudFront, it will be provided to the browser.
- The browser caches the content received from CloudFront and uses it from the next time.
- If CloudFront’s cache also needs to be updated(max-age), first provide the cache of V1 with stale-while-revalidate to the browser, and then update the cache by fetching content from the actual server in the background.
Completed
Done! We’ve seen what web cache is and how to apply cache when providing web services with static files using AWS S3 and CloudFront.
I hope this blog post helps you understand web cache and how to apply cache in AWS.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.