Outline
recently, many people use Micro-interactions to make the app animations. in this blog, we will see how to apply the animation created by Adobe After Effects, to RN(React Native) project by using Airbnb’s lottie
library.
- lottie official site: https://airbnb.design/lottie/
Installation
execute below command to install lottie-react-native
library to use lottie
library on RN(React Native).
Upper 0.60
npm install --save lottie-react-native lottie-ios
Connect Library
After installing, execute the command below to connect the library on iOS.
cd ios
pod install
cd ..
Under 0.59
npm install --save lottie-react-native
Link Library
after installing lottie-react-native
to use lottie
on RN(React Native), link the library to follow OS specific instruction.
- iOS
execute below command to link lottie-react-native
library to RN(React Native) for using lottie
on iOS.
react-native link lottie-ios
react-native link lottie-react-native
and open ios/[project_name].xcodeproj
or ios/[project_name].xcworkspace
file to execute xcode. and then configure additional settings like below.
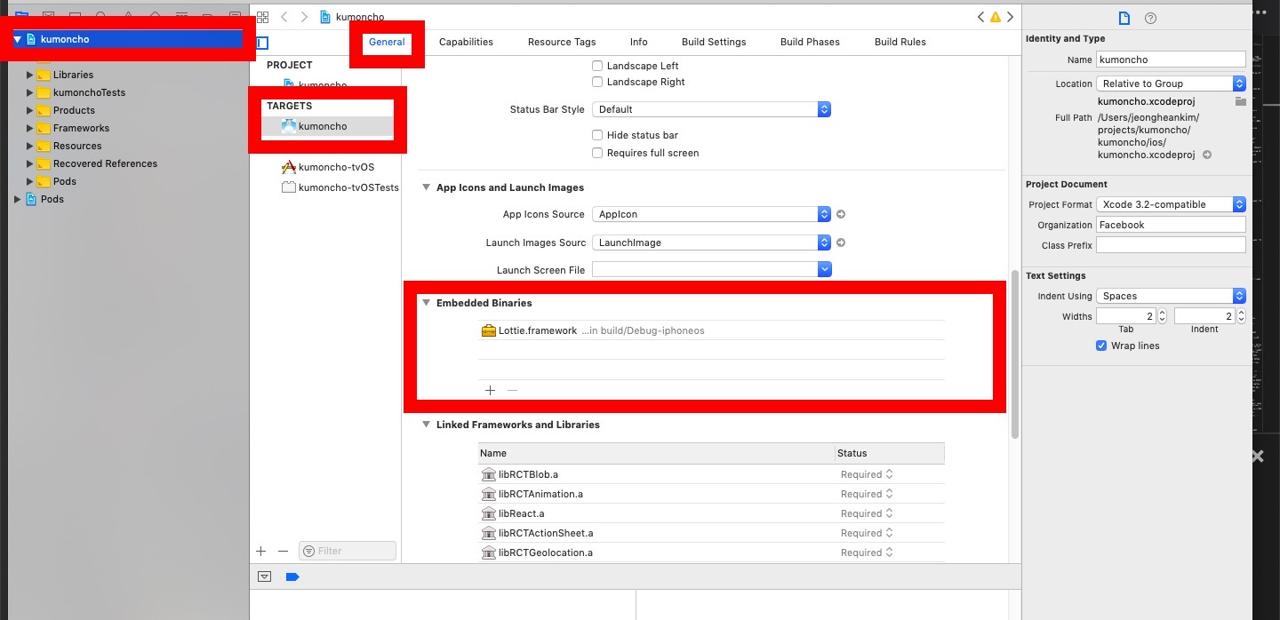
- select the project name on left side menu.
- select the project name on
TARGETS
- select
General
on the top of the screen. - scroll down and you can see
Embedded Binaries
section. click+
button and searchLottie.framework
. selectios
and add.
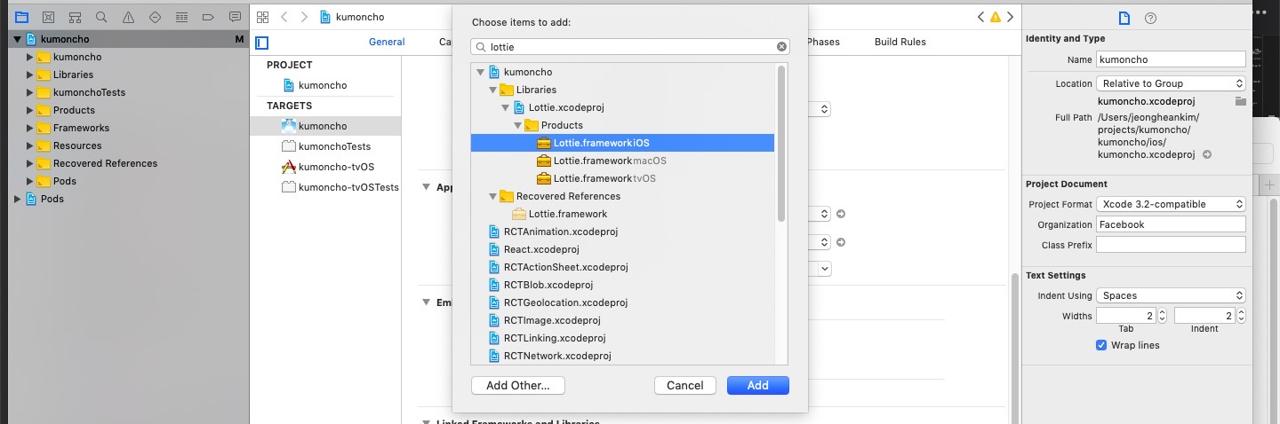
- Android
if you did above iOS
procedure, you don’t need to do anything to connect lottie
library for Android. when below command was executed, Android was connected to lottie-react-native
.
react-native link lottie-react-native
How TO Use
we need Adobe After Effects animation file to use lottie on RN(React Native). we won’t talk about how to make lottie animation file by Adobe After Effects in this blog. if you want to know how to make lottie animation file by Adobe After Effects, see below link.
or click below link to search the Micro-interactions what you need.
the animation file by Adobe After Effects or you downloaded is json
file type.
below code is how to use lottie on RN(React Native) project for applying Micro-interactions
...
import LottieView from 'lottie-react-native';
...
export default class BasicExample extends React.Component {
render() {
return (
<LottieView
source={require('./animation.json')}
autoPlay
loop
/>
);
}
}
...
Animation File with Images
when you create the animation using Adobe After Effects, sometimes, you need to export the animation file that includes images.
if you export the animation file that includes images, you can see data.json
like below.
// data.json
{
...
"assets": [
{
"id": "image_0",
"w": 588,
"h": 792,
"u": "images/",
"p": "main_character.jpg",
"e": 0
}
]
...
we need to add images in the animation file to the native platforms.
- iOS
execute xcode to select ios/[project name].xcworkspace
(or ios/xcodeproj
) in RN(React Native) project.
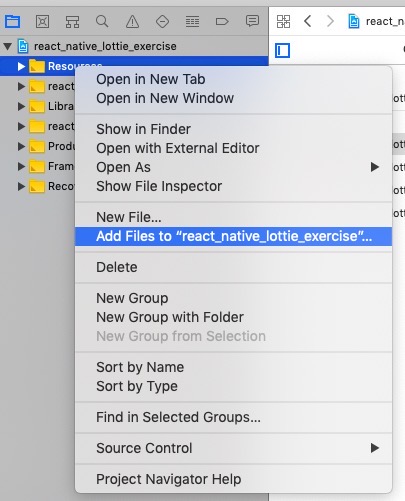
right click Resources
folder below the project name on left side menu, and select Add Files to [project name]
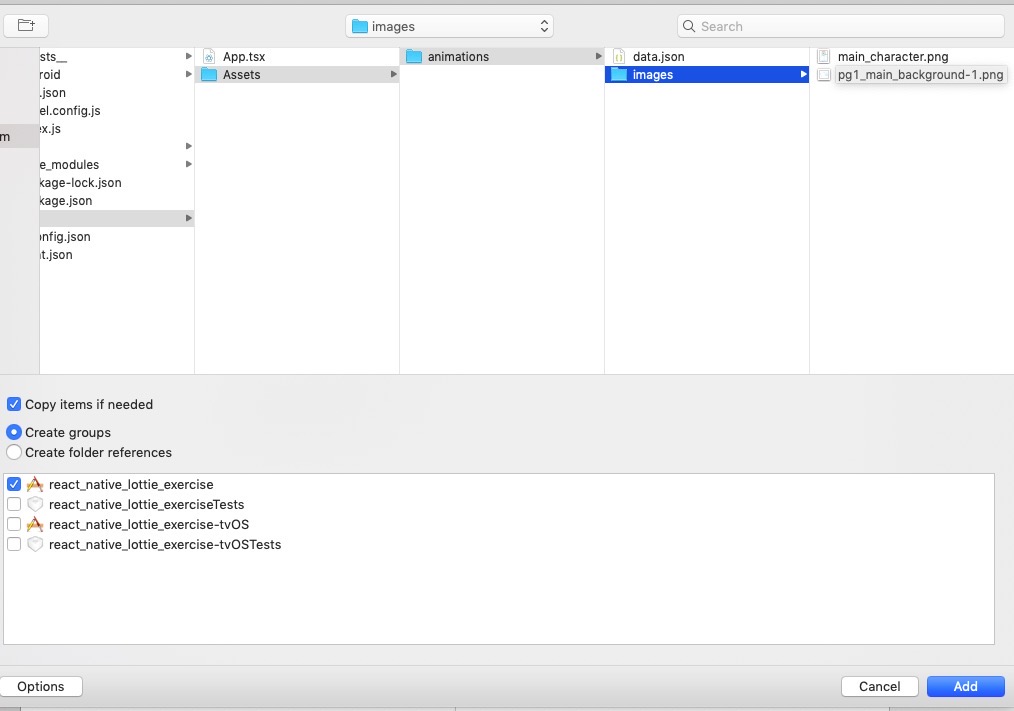
select image files what you want to add, select Copy items if needed
options on below and add.
if you can’t see Resources
folder, right click the project name on left side menu, and select New Group without Folder
. after adding the group, rename it to Resources
.
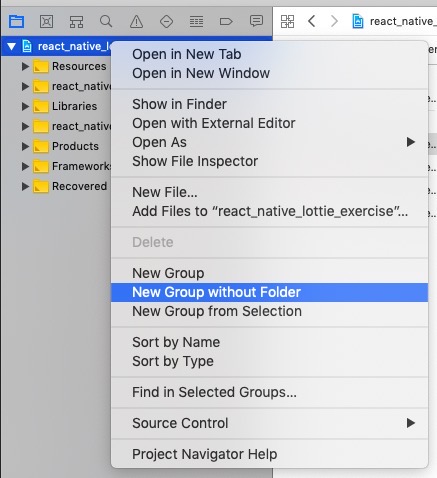
- Android
Android is more simple than iOS. create the folder that you copy images on android/app/src/main/assets
in RN(React Native) project. in here, I created images
folder. and then, copy images to that folder(android/app/src/main/assets/images
).
after copying, modify the source code adding imageAssetsFolder={'images'}
like below.
<LottieView
source={require('./animation.json')}
autoPlay
loop
imageAssetsFolder={'images'}
/>
Git Repository
we created git repository about how to use Lottie
in this case. you can click the link below to check the example.
- git repository: react_native_lottie_exercise
Fix Error
(0.60 / iOS) ld: warning: Could not find or use auto-linked library
When I executed the command below,
react-native run-ios
I got the error message like below.
ld: warning: Could not find or use auto-linked library ‘swiftCoreFoundation’
ld: warning: Could not find or use auto-linked library ‘swiftCompatibility50’
ld: warning: Could not find or use auto-linked library ‘swiftSwiftOnoneSupport’
ld: warning: Could not find or use auto-linked library ‘swiftObjectiveC’
ld: warning: Could not find or use auto-linked library ‘swiftQuartzCore’
ld: warning: Could not find or use auto-linked library ‘swiftCore’
ld: warning: Could not find or use auto-linked library ‘swiftCoreGraphics’
ld: warning: Could not find or use auto-linked library ‘swiftDispatch’
ld: warning: Could not find or use auto-linked library ‘swiftDarwin’
ld: warning: Could not find or use auto-linked library ‘swiftUIKit’
ld: warning: Could not find or use auto-linked library ‘swiftCompatibilityDynamicReplacements’
ld: warning: Could not find or use auto-linked library ‘swiftCoreImage’
ld: warning: Could not find or use auto-linked library ‘swiftFoundation’
ld: warning: Could not find or use auto-linked library ‘swiftMetal’
To solve it, execute /ios/[project name].xcworkspace
file to open Xcode.
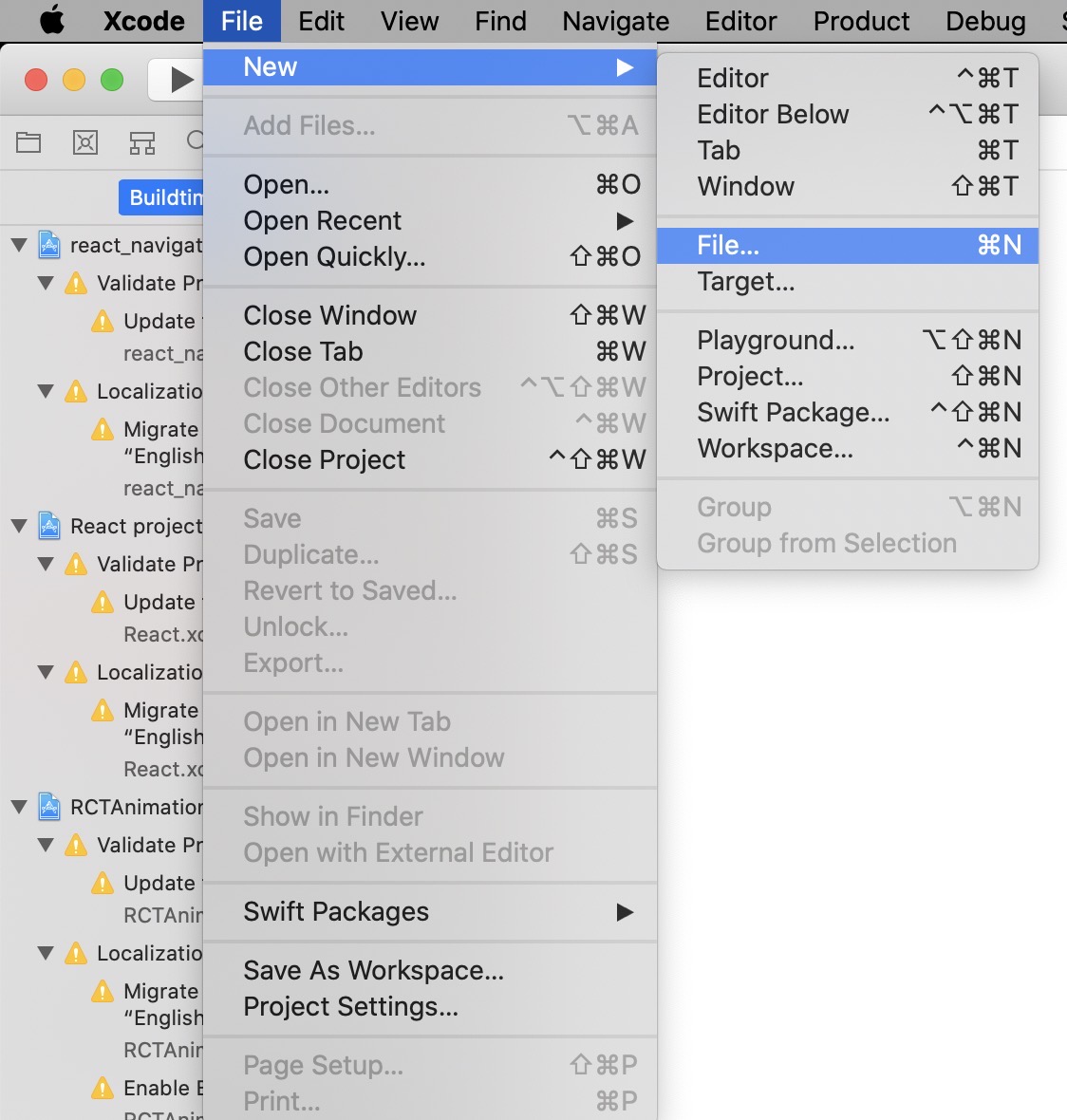
Select File > New > File...
on the screen like above.
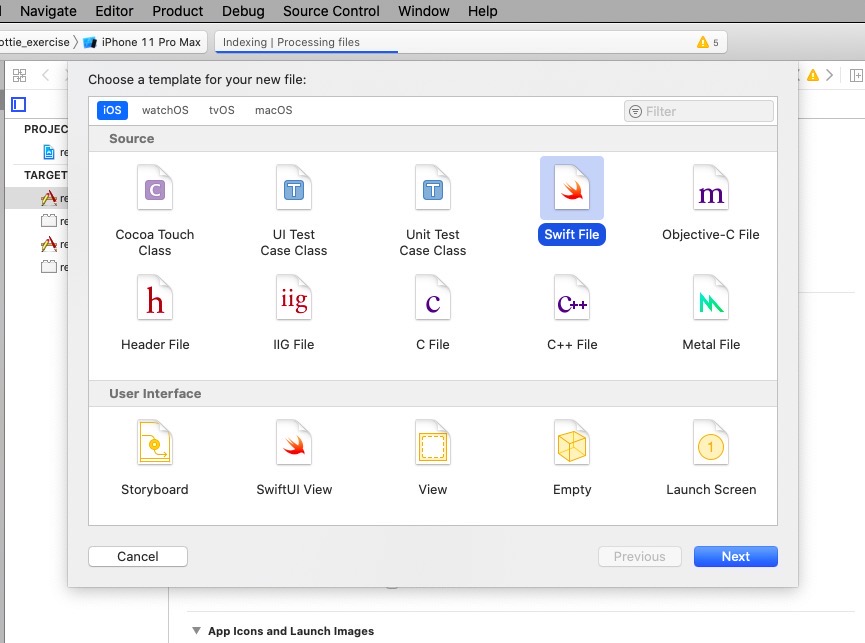
on the screen above, Select Swift File
and select Next
button on the right bottom.
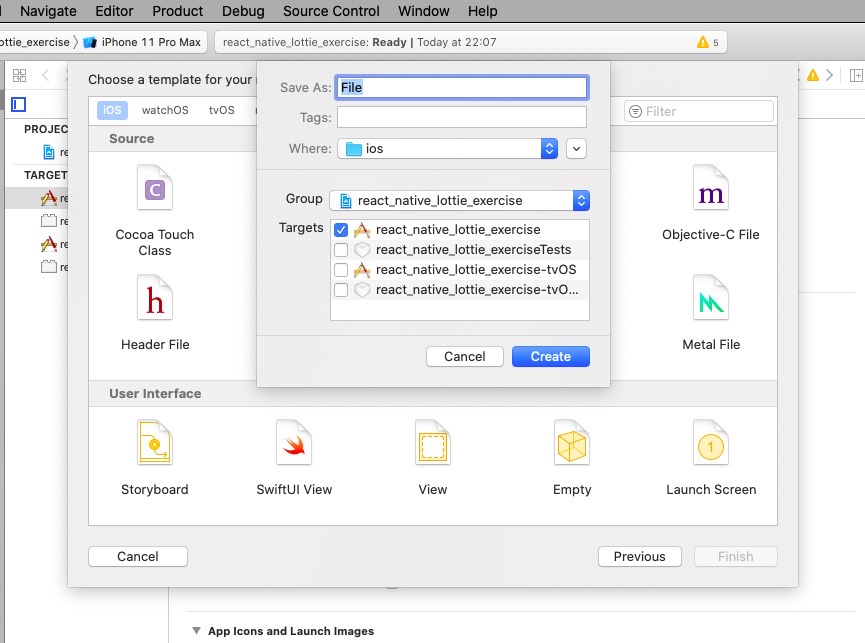
Select Create
button on the create new file screen like above.
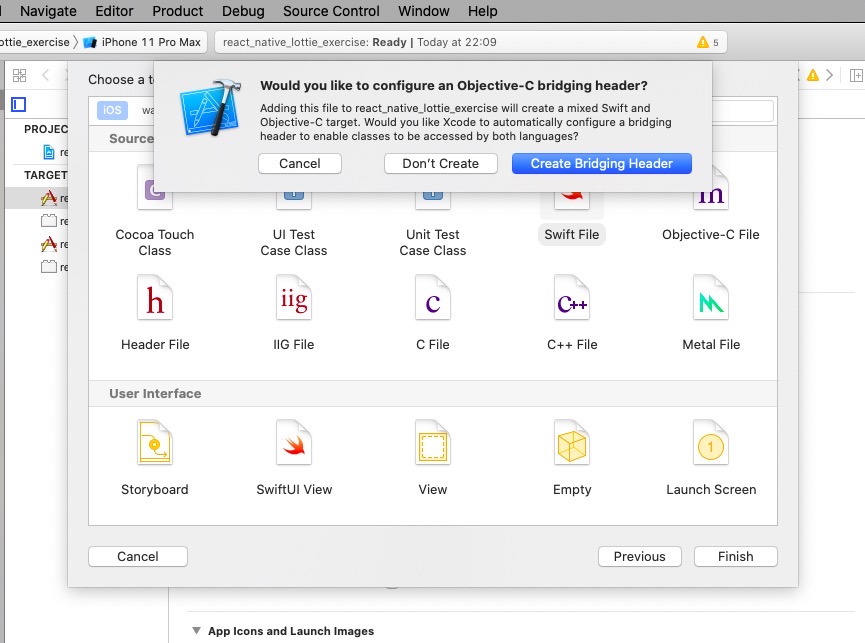
Select Create Bridging Header
button on the right bottom of the screen like above.
And then, execute the command below to delete the previous project in iOS simulator.
rm -rf ~/Library/Developer/Xcode/DerivedData/*
Lastly, select Product > Clean Build Folder
on the screen like below.
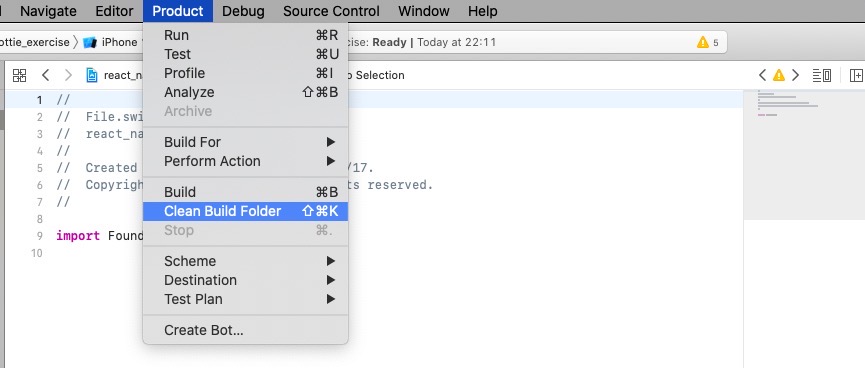
Now, execute the command below to execute iOS.
react-native run-ios
error: Cycle in dependencies between targets
I’ve used react-native-lottie
well after implementing on RN(React Native), but after installing another library, when I built my RN(React Native) project, I got the error like below.
Build system information
error: Cycle in dependencies between targets 'LottieLibraryIOS' and 'LottieReactNative'; building could produce unreliable results.
Cycle path: LottieLibraryIOS → LottieReactNative → LottieLibraryIOS
Cycle details:
...
I deleted Pods
folder and node_modules
folder, and installed again, but the error was still occurred.
below is how to fix the error in my case. if you have same issue as I have, try to do below.
execute ios/[project_name].xcworkspace
file in RN(React Native) project to open xcode.
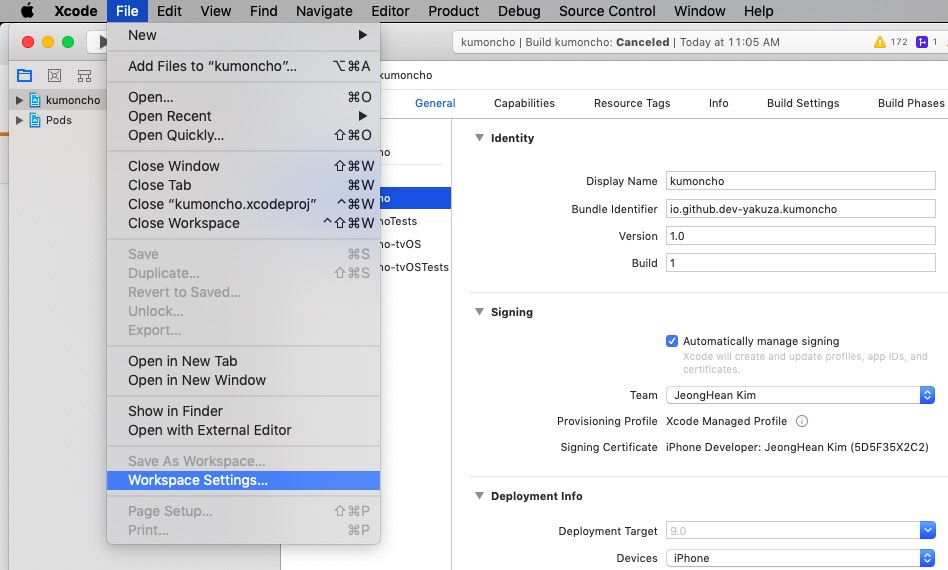
after executing xcode, click File > Workspace Settings...
like above.
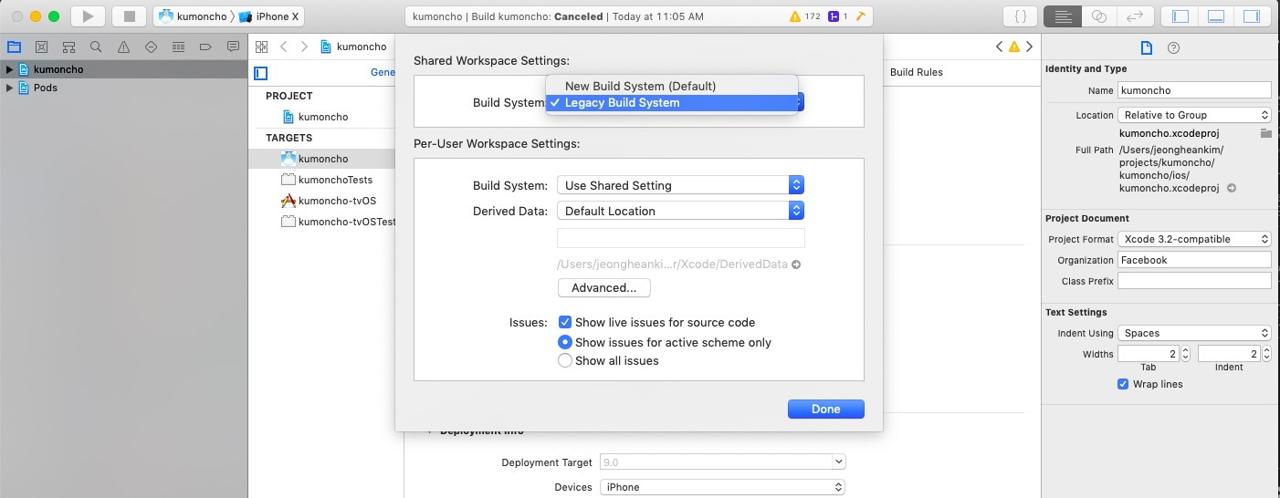
change the Build System to Legacy Build System
from New Build System (Default)
.
in my case, after changing the Build System, I can build successfully. I hope this way is helpful to you.
Android Build Error
when you build on Android, sometimes, you can see the error like below.
Execution failed for task ':app:transformClassesWithDexBuilderForDevDebug'.
in this case, I modified android/app/build.gradle
file in RN(React Native) project like below.
android{
...
configurations.all {
resolutionStrategy {
force 'com.airbnb.android:lottie:2.5.5'
}
}
}
- Reference: Java 8 compilation error version 2.5.6
Completed
we’ve done to use lottie on RN(React Native) to show Micro-interactions. it’s quite simple, isn’t it? I think it’s more difficult to make the animation than applying it by lottie. I don’t know how to use After Effects, so I use https://lottiefiles.com/ site. I recommend for you to use lottie to use Micro-interactions on you project.
Reference
- lottie official site: https://airbnb.design/lottie/
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.