Content
Outline
In this blog post, we’ll see how to get current geolocation in React Native. To get location information, we’ll use react-native-geolocation-service
library. You can see the detail about react-native-geolocation-service library via the link below.
There is a source code about this blog post. If you want to see the source code, check the link below.
The example source code is based on the libraries below. If you want to know the details, check the links below.
How to install react-native-geolocation-service
Execute the link below to install react-native-geolocation-service.
npm install --save react-native-geolocation-service
Link the library
we need to link the library to React Native project.
Version >= 0.60
Execute the commend below to link the library on iOS.
cd ios
pod install
To use react-native-geolocation-service You need to make iOS project to support Swift. To make Swift supported, execute ios/[project name]xcworkspace
file to open Xcode.
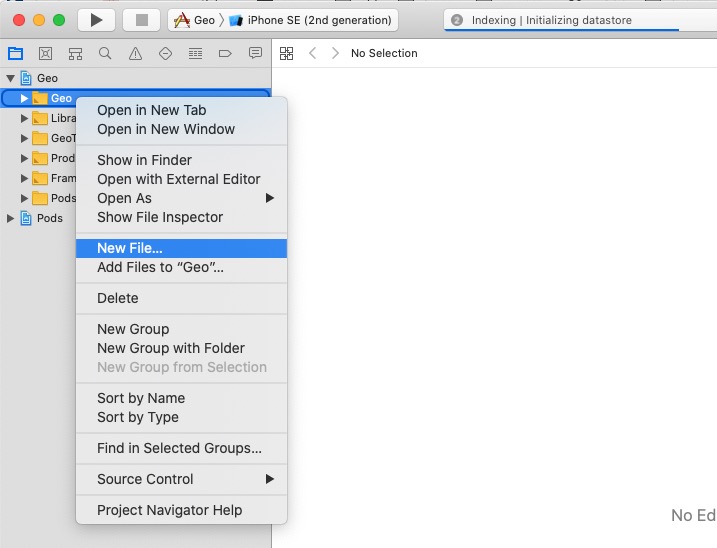
After Xcode is executed, right-click the folder on the top left, and click New File...
menu.
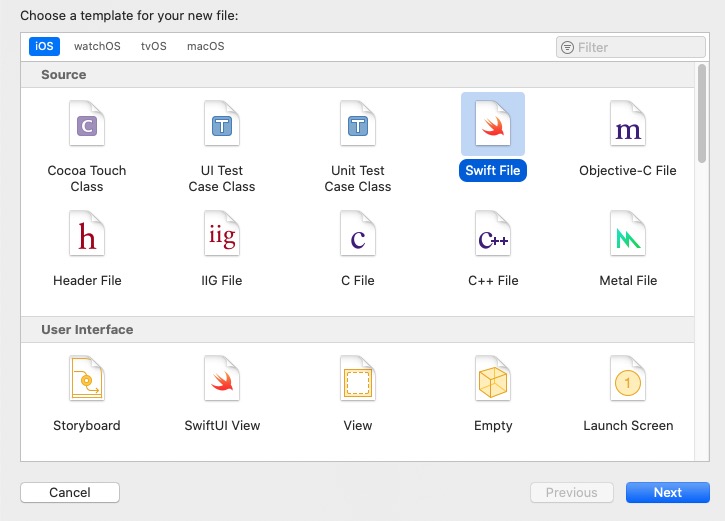
On the screen above, click Swift File
and click Next
on the right bottom.
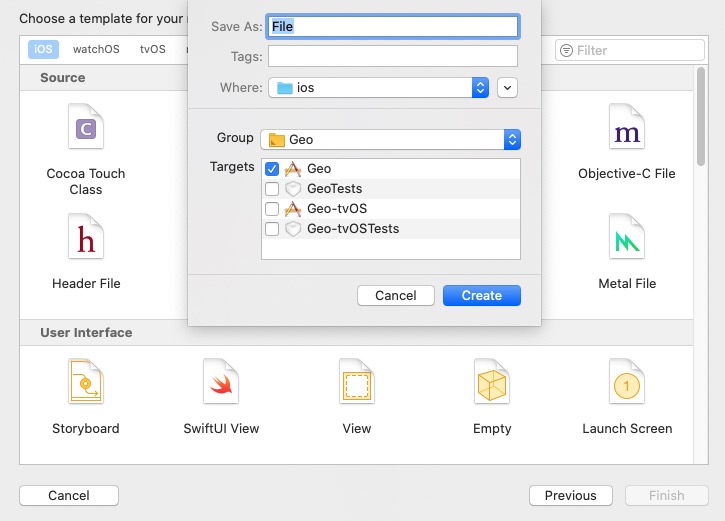
Insert the file name whatever you want, and click Create
button on the right bottom.
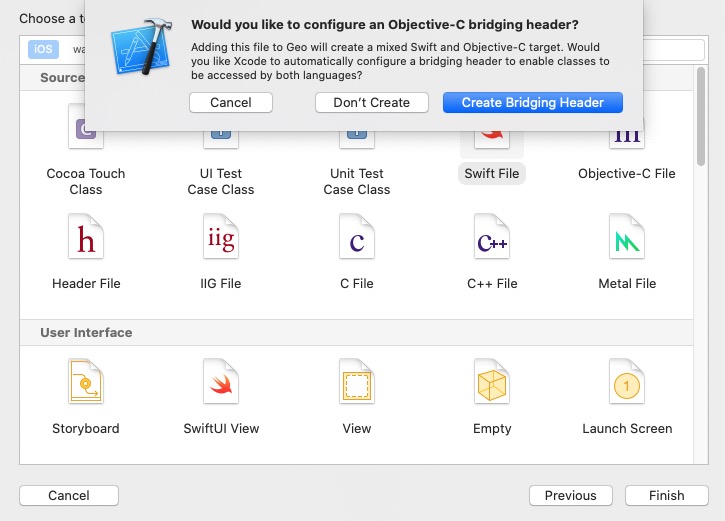
On the screen above, click Create Bridging Header
on the right bottom to make Swift supported.
Version <= 0.59
If you use React Native version <= 0.59, see the official site links below.
Configure Permission
Configure iOS Permission
To use the geolocation information on iOS, we need to configure the permissions like below in ios/[Project Name]/info.plist
file.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
...
<key>NSLocationWhenInUseUsageDescription</key>
<string>Program requires GPS to track cars and job orders</string>
<key>NSLocationAlwaysUsageDescription</key>
<string>Program requires GPS to track cars and job orders</string>
<key>NSLocationAlwaysAndWhenInUseUsageDescription</key>
<string>Program requires GPS to track cars and job orders</string>
...
</dict>
</plist>
If your app uses the geolocation on the foreground only, you need to set NSLocationWhenInUseUsageDescription
only.
If your app needs to get the geolocation on the background, you also need to configure NSLocationAlwaysUsageDescription
and NSLocationAlwaysAndWhenInUseUsageDescription
, and set Location updates
on Background Modes
like below.
To get the geolocation on the background, open Xcode and click + Capability
like below.
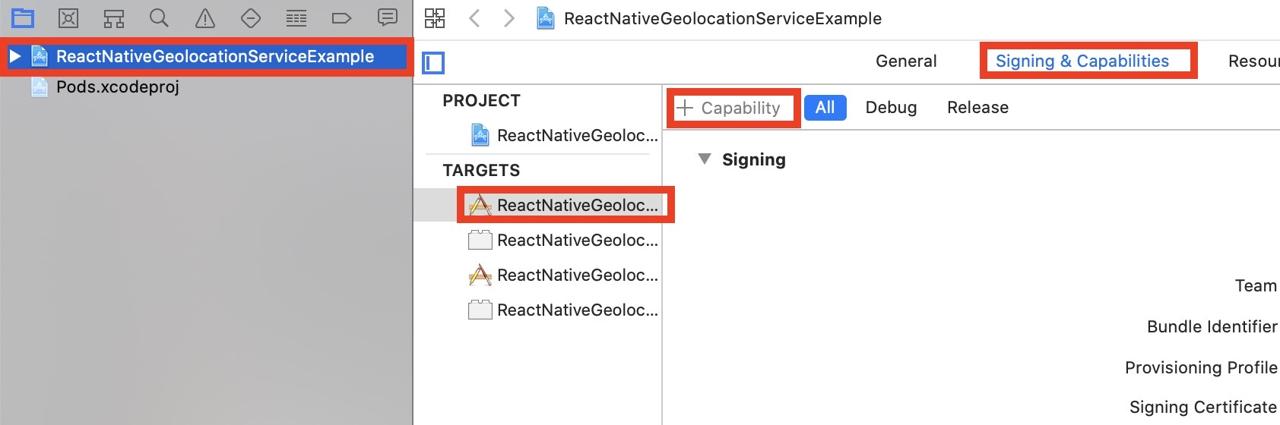
When the Capability
search screen is shown up, search Background Modes
and double-click the result to add it.
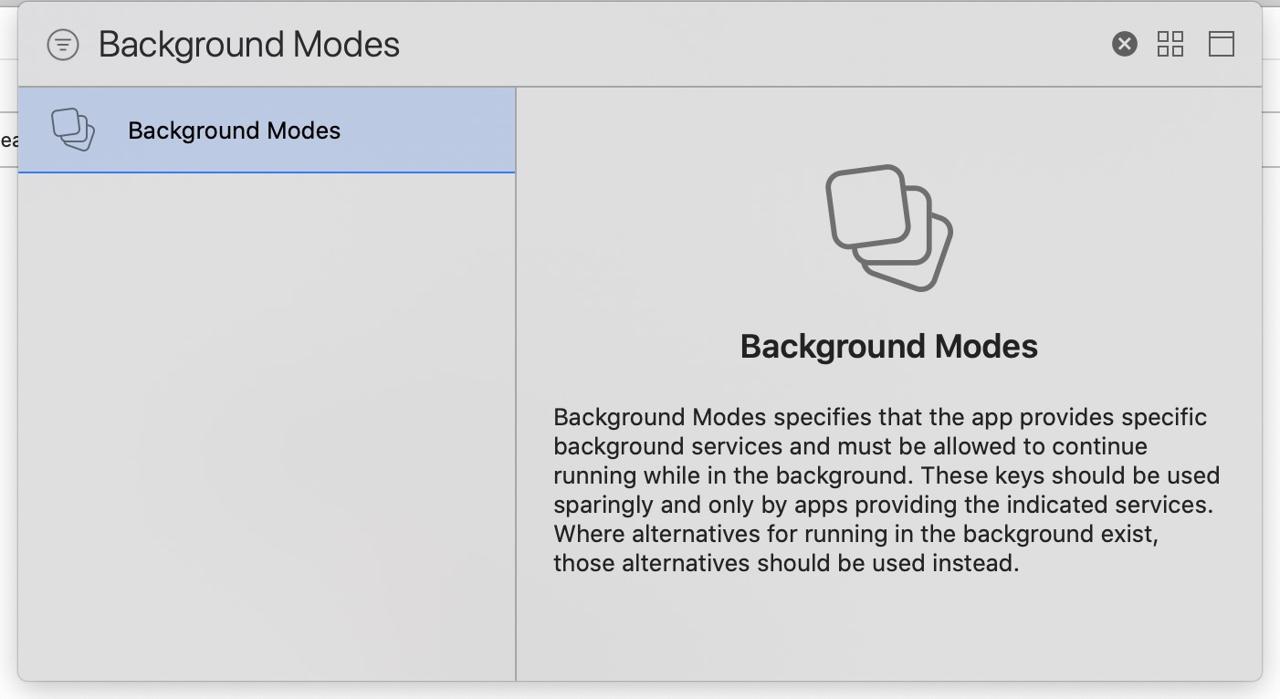
After adding Background Modes
, click Location updates
on the Background Modes
.
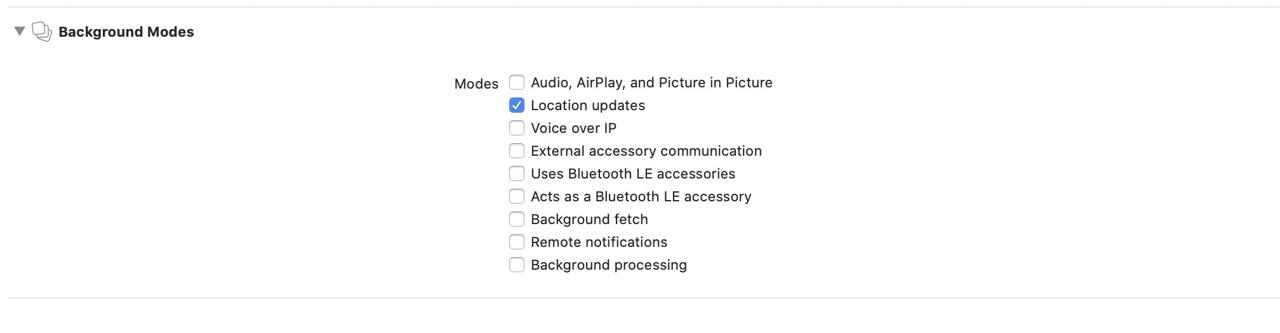
After configuration, you should use the code below to get the permission.
import {Platform} from 'react-native';
import Geolocation from 'react-native-geolocation-service';
...
useEffect(() => {
if (Platform.OS === 'ios') {
Geolocation.requestAuthorization('always');
}
}, []);
...
Configure Android Permission
Open android/app/src/main/AndroidManifest.xml
file and modify it like below to get user location information on Android.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.reactnativegeolocationserviceexample">
...
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" />
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
...
<application
How to use
we can get the geolocation to use react-native-geolocation library like below.
How to get current geolocation
The code below is about how to get current geolocation.
Geolocation.getCurrentPosition(
(position) => {
console.log(position);
},
(error) => {
// See error code charts below.
console.log(error.code, error.message);
},
{ enableHighAccuracy: true, timeout: 15000, maximumAge: 10000 }
);
The example below is the full source code about how to get current geolocation.
import React, {useState, useEffect} from 'react';
import Styled from 'styled-components/native';
import Geolocation from 'react-native-geolocation-service';
const Container = Styled.View`
flex: 1;
justify-content: center;
align-items: center;
`;
const Label = Styled.Text`
font-size: 24px;
`;
interface ILocation {
latitude: number;
longitude: number;
}
const CurrentPosition = () => {
const [location, setLocation] = useState<ILocation | undefined>(undefined);
useEffect(() => {
Geolocation.getCurrentPosition(
position => {
const {latitude, longitude} = position.coords;
setLocation({
latitude,
longitude,
});
},
error => {
console.log(error.code, error.message);
},
{enableHighAccuracy: true, timeout: 15000, maximumAge: 10000},
);
}, []);
return (
<Container>
{location ? (
<>
<Label>Latitude: {location.latitude}</Label>
<Label>Latitude: {location.longitude}</Label>
</>
) : (
<Label>Loading...</Label>
)}
</Container>
);
};
export default CurrentPosition;
You can see the result like below if use the source code above.
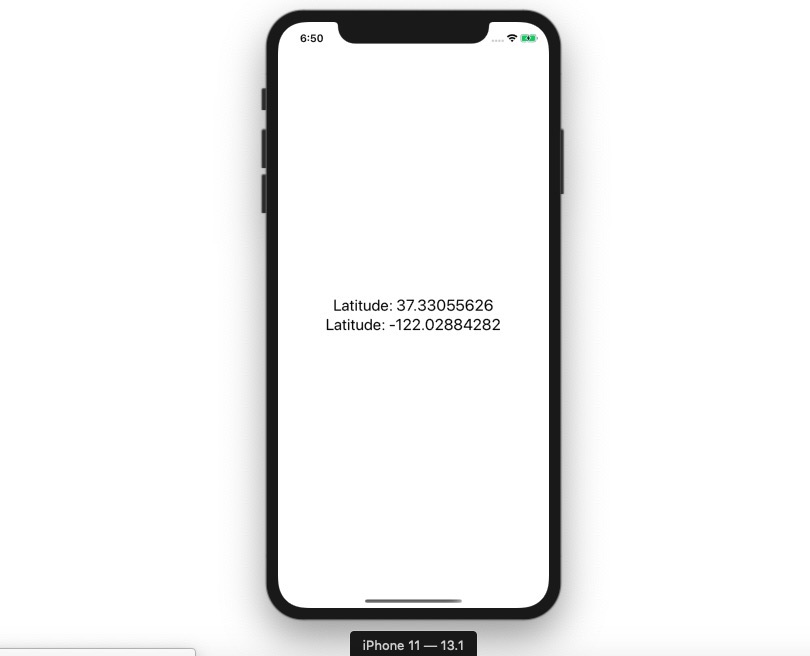
How to track user geolocation
We can not only get user current location, but also track user geolocation by react-native-geolocation-service.
The source code below is about how to track user geolocation.
_watchId = Geolocation.watchPosition(
position => {
const {latitude, longitude} = position.coords;
setLocation({latitude, longitude});
},
error => {
console.log(error);
},
{
enableHighAccuracy: true,
distanceFilter: 0,
interval: 5000,
fastestInterval: 2000,
},
);
After tracking, we can stop it by the code below.
if (_watchId !== null) {
Geolocation.clearWatch(_watchId);
}
The example below is the full source code about how to track user geolocation.
import React, {useState, useEffect} from 'react';
import Styled from 'styled-components/native';
import Geolocation from 'react-native-geolocation-service';
const Container = Styled.View`
flex: 1;
justify-content: center;
align-items: center;
`;
const Label = Styled.Text`
font-size: 24px;
`;
interface ILocation {
latitude: number;
longitude: number;
}
const WatchLocation = () => {
const [location, setLocation] = useState<ILocation | undefined>(undefined);
useEffect(() => {
const _watchId = Geolocation.watchPosition(
position => {
const {latitude, longitude} = position.coords;
setLocation({latitude, longitude});
},
error => {
console.log(error);
},
{
enableHighAccuracy: true,
distanceFilter: 0,
interval: 5000,
fastestInterval: 2000,
},
);
return () => {
if (_watchId) {
Geolocation.clearWatch(_watchId);
}
};
}, []);
return (
<Container>
{location ? (
<>
<Label>Latitude: {location.latitude}</Label>
<Label>Latitude: {location.longitude}</Label>
</>
) : (
<Label>Loading...</Label>
)}
</Container>
);
};
export default WatchLocation;
You can use the simulator for testing to track user location information. Open Debug > Location
menu and choose one of City Run, City Bicycle Ride, Freeway Drive
menu like the screen below. You can see the location changed.
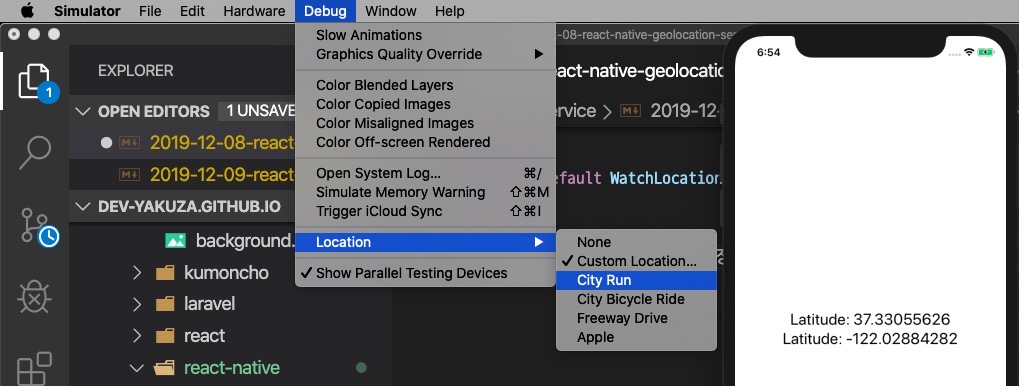
Completed
We’ve seen how to get user location information by react-native-geolocation-service. Also, we’ve seen how to track user geolocation by watchPosition
.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.