Contents
Outline
When you develop an app with Flutter, sometimes you want to implement a feature that scrolls the entire screen like TikTok
. In this case, you can easily implement it using the PageView
widget provided by Flutter.
In this blog post, I will introduce how to use the PageView
widget.
You can see the full source code introduced in this blog post at the link below.
PageView widget
The PageView
widget provided by Flutter allows you to scroll the entire screen. You can set up pages by passing multiple widgets through the children
property.
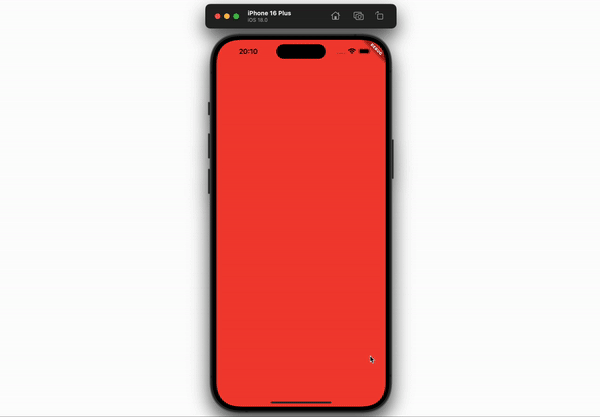
You can see the details of the PageView
widget in the following official document.
- Official document: PageView class
How to use PageView
The PageView
widget is basically provided by Flutter, so you can easily use the PageView
widget as follows.
class MyHomePage extends StatelessWidget {
MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: PageView(
children: [
Container(
width: double.infinity,
height: double.infinity,
color: Colors.red,
),
Container(
width: double.infinity,
height: double.infinity,
color: Colors.blue,
),
Container(
width: double.infinity,
height: double.infinity,
color: Colors.green,
),
],
),
);
}
}
When you set the widgets you want to display on the screen in the children
of the PageView
widget, you can scroll the entire screen to move between pages like the following.
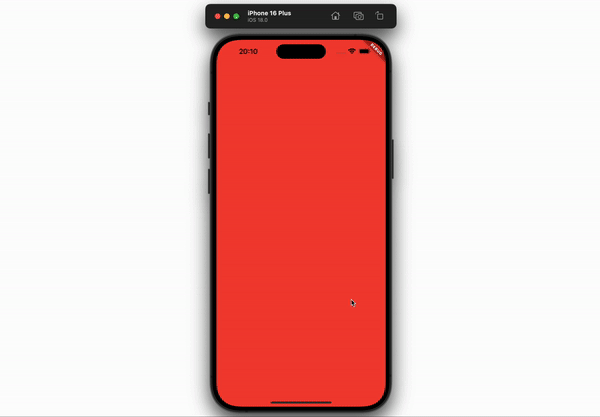
In here, I use the Container
widget to easily check the implementation.
PageController
You can use the PageController
to determine the initial page to display.
class MyHomePage extends StatelessWidget {
final pageController = PageController(
initialPage: 1,
);
MyHomePage({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
body: PageView(
controller: pageController,
children: [
...
],
),
);
}
}
You can set the index of the page to be displayed initially in the initialPage
property of the PageController
. After modifying the code like this and running the app again, you can see that the initially displayed page has changed.
scrollDirection property
You can set the scroll direction using the scrollDirection
property of the PageView
widget. By default, the scrollDirection
property is set to Axis.horizontal
, but you can set it to Axis.vertical
to scroll vertically.
PageView(
controller: pageController,
scrollDirection: Axis.vertical,
children: [
...
],
)
When you modify the code like this, you can scroll vertically like the following.
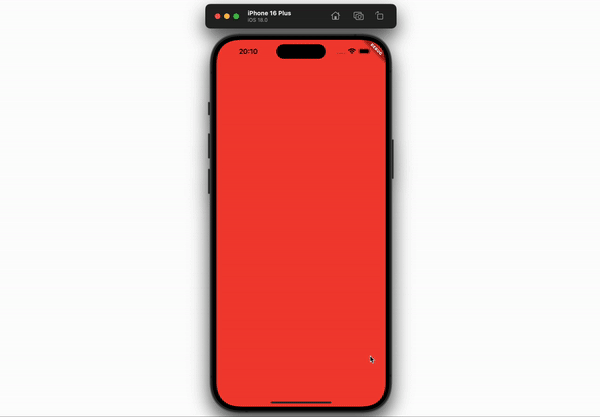
onPageChanged property
You can set a callback function that is called when the page is changed using the onPageChanged
property of the PageView
widget. This allows you to perform additional tasks when the page is changed.
PageView(
controller: pageController,
onPageChanged: (index) {
print('Page changed to $index');
},
children: [
...
],
)
PageView Builder
You can dynamically create pages using the PageView.builder
constructor of the PageView
widget. You can pass a function that creates pages through the itemBuilder
property.
PageView.builder(
controller: pageController,
itemCount: 10,
itemBuilder: (context, index) {
return Container(
width: double.infinity,
height: double.infinity,
color: index % 2 == 0 ? Colors.red : Colors.blue,
);
},
)
Completed
Done! We’ve seen how to scroll the entire page using the PageView
widget provided by Flutter. By using the PageView
widget, you can easily scroll the entire page, and you can set the scroll direction using the scrollDirection
property.
When you use the PageView
widget, you can easily implement a feature like the TikTok
app. If you need a feature that scrolls the entire screen in the app you are developing, try implementing it using the PageView
widget.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.