Outline
In this blog post, I will introduce how to open the web browser, send an email or call a phone number by url_launcher
in Flutter.
- url_launcher: https://pub.dev/packages/url_launcher
You can see full source code of this blog post on the link below.
Install url_launcher
To check how to use url_launcher
in Flutter, execute the following command to create a new Flutter project.
flutter create url_launcher_example
And then, execute the following command to install url_launcher
in the project.
flutter pub add url_launcher
Next, let’s see how to use url_launcher.
Configuration
To use url_launcher
, you need to configure the following.
iOS
To use url_launcher
in iOS, open the ios/Runner/Info.plist
file and modify it like the below.
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE plist PUBLIC "-//Apple//DTD PLIST 1.0//EN" "http://www.apple.com/DTDs/PropertyList-1.0.dtd">
<plist version="1.0">
<dict>
...
<key>LSApplicationQueriesSchemes</key>
<array>
<string>https</string>
<string>http</string>
</array>
</dict>
</plist>
Android
To use url_launcher
in Android, open the android/app/src/main/AndroidManifest.xml
file and modify it like the below.
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.url_launcher_example">
<queries>
<!-- If your app opens https URLs -->
<intent>
<action android:name="android.intent.action.VIEW" />
<data android:scheme="https" />
</intent>
<!-- If your app makes calls -->
<intent>
<action android:name="android.intent.action.DIAL" />
<data android:scheme="tel" />
</intent>
<!-- If your sends SMS messages -->
<intent>
<action android:name="android.intent.action.SENDTO" />
<data android:scheme="smsto" />
</intent>
<!-- If your app sends emails -->
<intent>
<action android:name="android.intent.action.SEND" />
<data android:mimeType="*/*" />
</intent>
</queries>
<application>
...
</application>
</manifest>
How to use
After installing and configuring the url_launcher
, you can use the launchUrl
function to open the web browser like the below.
import 'package:url_launcher/url_launcher.dart';
...
launchUrl(
Uri.parse('https://deku.posstree.com/en/'),
);
As you can see, you can generate the Uri
, which is used in launchUrl
function, with the string. And also, you can generate it with the constructor like the below.
// https://play.google.com/store/apps/details?id=com.google.android.tts
Uri(
scheme: 'https',
host: 'play.google.com',
path: 'store/apps/details',
queryParameters: {"id": 'com.google.android.tts'},
),
Also, you can use the canLaunchUrl
function to check the URL can be executed or not.
const url = Uri.parse('https://deku.posstree.com/en/');
if (await canLaunchUrl(url)) {
launchUrl(url);
}
And, you can use the following URL to open the other apps.
- Email:
launchUrl(Uri.parse('mailto:[email protected]?subject=Hello&body=Test'));
- Tel:
launchUrl(Uri.parse('tel:+1 555 010 999'));
- SMS:
launchUrl(Uri.parse('sms:5550101234'));
- Google Play store App page:
launchUrl(Uri.parse('http://play.google.com/store/apps/details?id=com.google.android.tts'));
Example
To check how to use url_launcher
in Flutter, open the lib/main.dart
file and modify it like the below.
import 'package:flutter/material.dart';
import 'package:url_launcher/url_launcher.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('URL Launcher'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: () async {
final url = Uri.parse(
'https://deku.posstree.com/en/',
);
if (await canLaunchUrl(url)) {
launchUrl(url);
} else {
// ignore: avoid_print
print("Can't launch $url");
}
},
child: const Text('Web Link'),
),
ElevatedButton(
onPressed: () async {
final url = Uri(
scheme: 'mailto',
path: '[email protected]',
query: 'subject=Hello&body=Test',
);
if (await canLaunchUrl(url)) {
launchUrl(url);
} else {
// ignore: avoid_print
print("Can't launch $url");
}
},
child: const Text('Mail to'),
),
ElevatedButton(
onPressed: () async {
final url = Uri.parse('tel:+1 555 010 999');
if (await canLaunchUrl(url)) {
launchUrl(url);
} else {
// ignore: avoid_print
print("Can't launch $url");
}
},
child: const Text('Tel'),
),
ElevatedButton(
onPressed: () async {
final url = Uri.parse('sms:5550101234');
if (await canLaunchUrl(url)) {
launchUrl(url);
} else {
// ignore: avoid_print
print("Can't launch $url");
}
},
child: const Text('SMS'),
),
],
),
),
);
}
}
When you press the button on the screen, you can see the app is opened depending on the URL.
Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: () async {
final url = Uri.parse(
'https://deku.posstree.com/en/',
);
if (await canLaunchUrl(url)) {
launchUrl(url);
} else {
// ignore: avoid_print
print("Can't launch $url");
}
},
child: const Text('Web Link'),
),
ElevatedButton(
onPressed: () async {
final url = Uri(
scheme: 'mailto',
path: '[email protected]',
query: 'subject=Hello&body=Test',
);
if (await canLaunchUrl(url)) {
launchUrl(url);
} else {
// ignore: avoid_print
print("Can't launch $url");
}
},
child: const Text('Mail to'),
),
ElevatedButton(
onPressed: () async {
final url = Uri.parse('tel:+1 555 010 999');
if (await canLaunchUrl(url)) {
launchUrl(url);
} else {
// ignore: avoid_print
print("Can't launch $url");
}
},
child: const Text('Tel'),
),
ElevatedButton(
onPressed: () async {
final url = Uri.parse('sms:5550101234');
if (await canLaunchUrl(url)) {
launchUrl(url);
} else {
// ignore: avoid_print
print("Can't launch $url");
}
},
child: const Text('SMS'),
),
],
),
Check
When you execute the code, you can see the following screen.
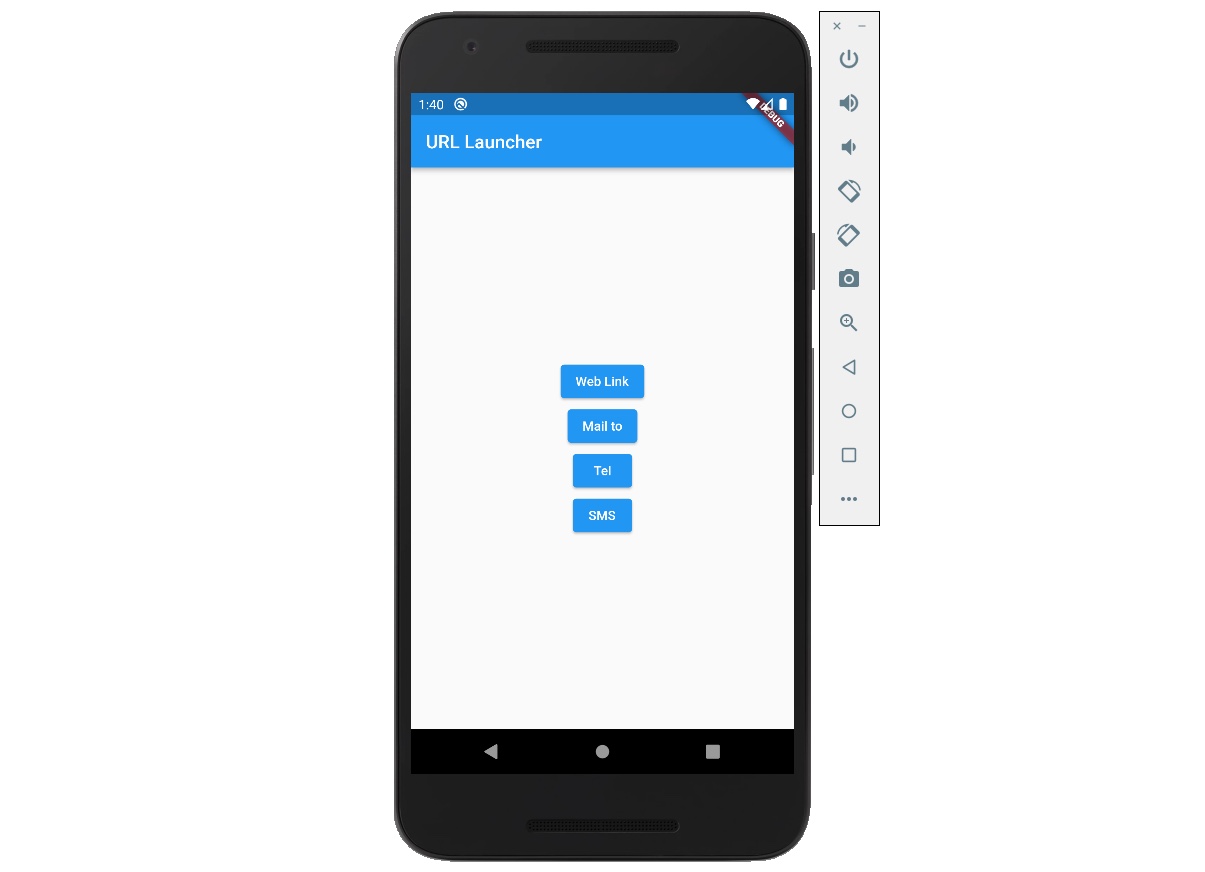
When you press the Web Link
button, you can see the web browser is opened.
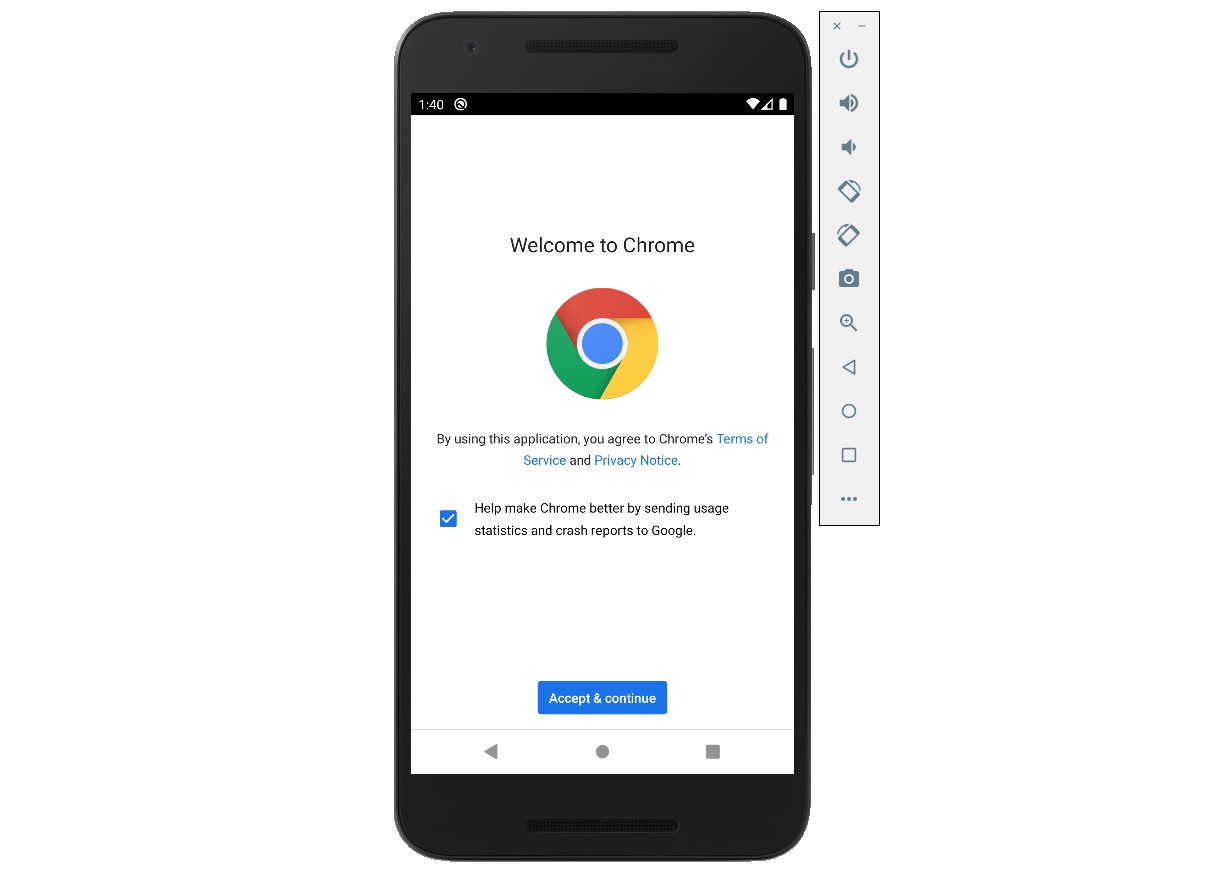
When you press the Mail to
button, you can see the email app is opened.
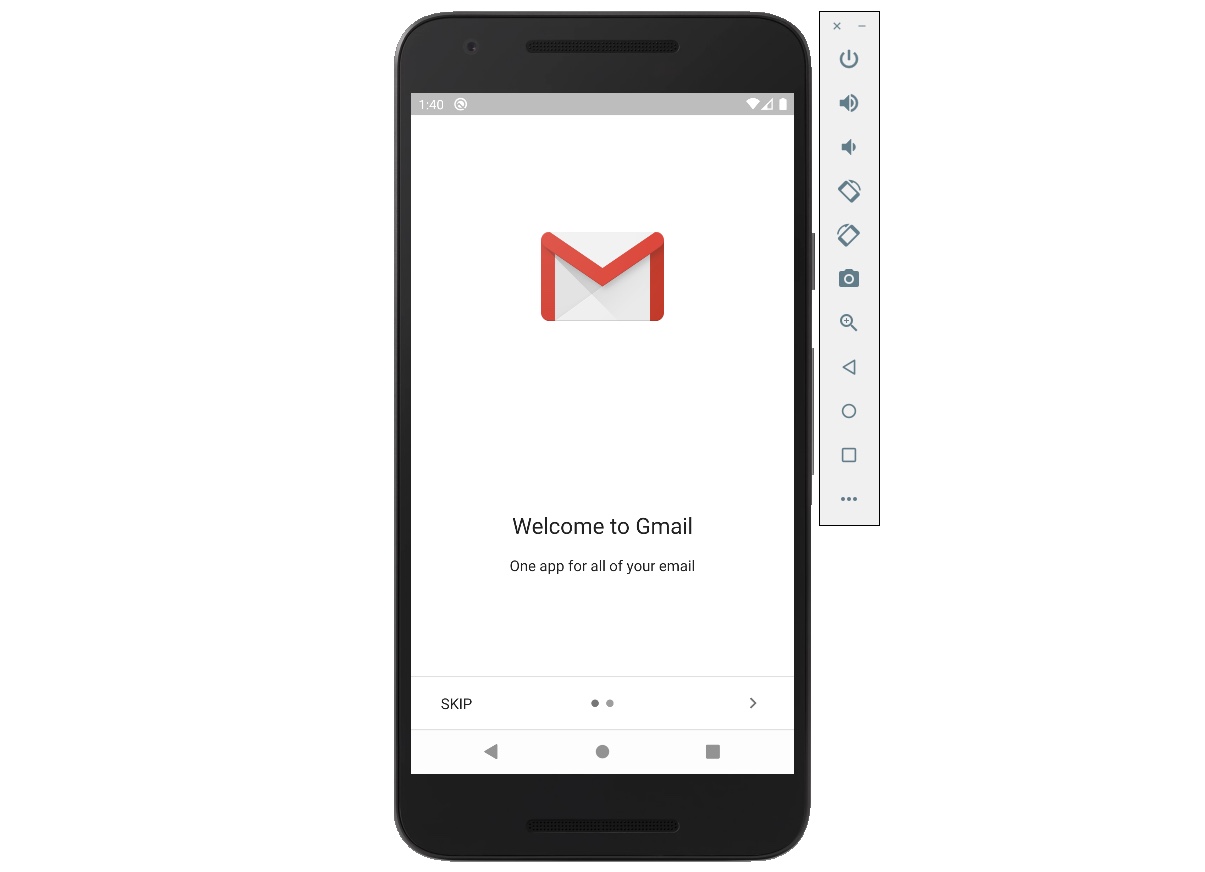
When you press the Tel
button, you can see the calling app is opened.
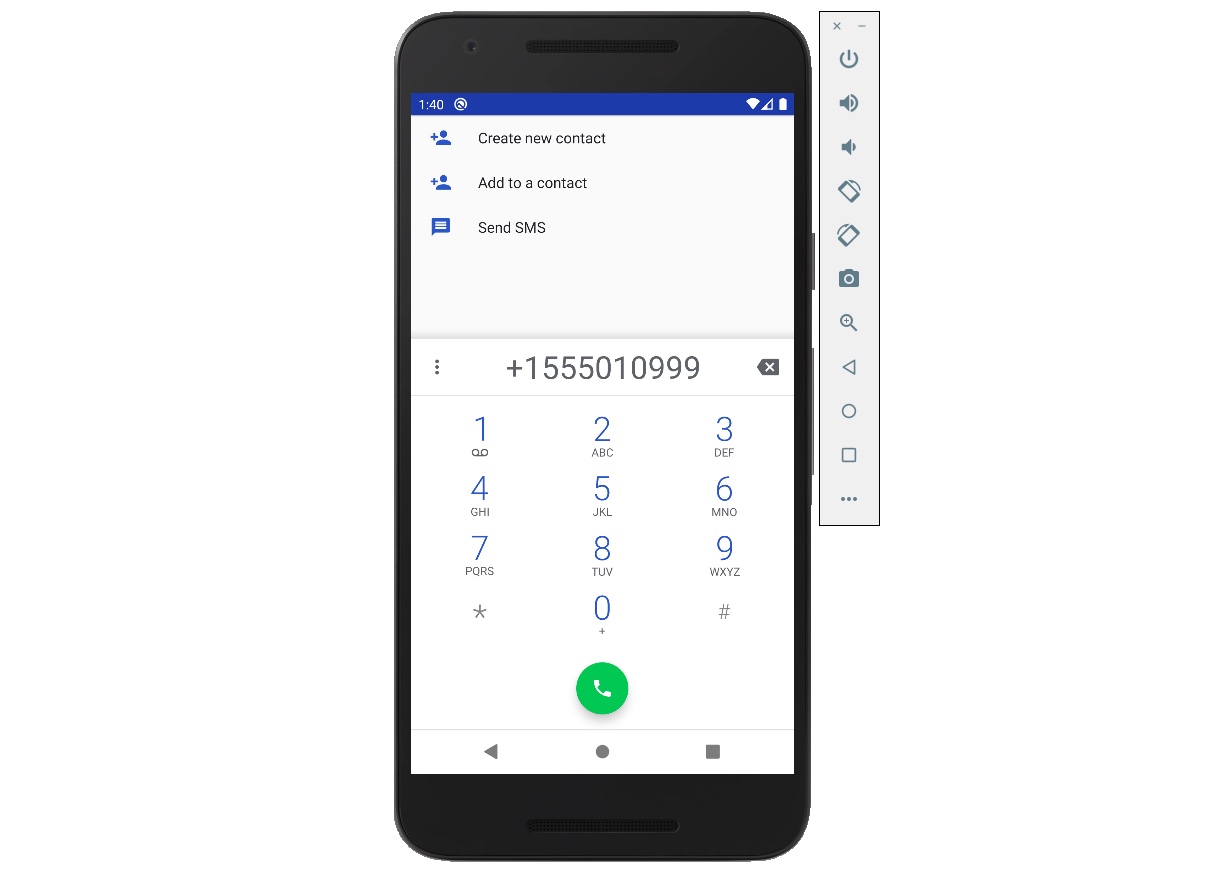
When you press the SMS
button, you can see the SMS app is opened.
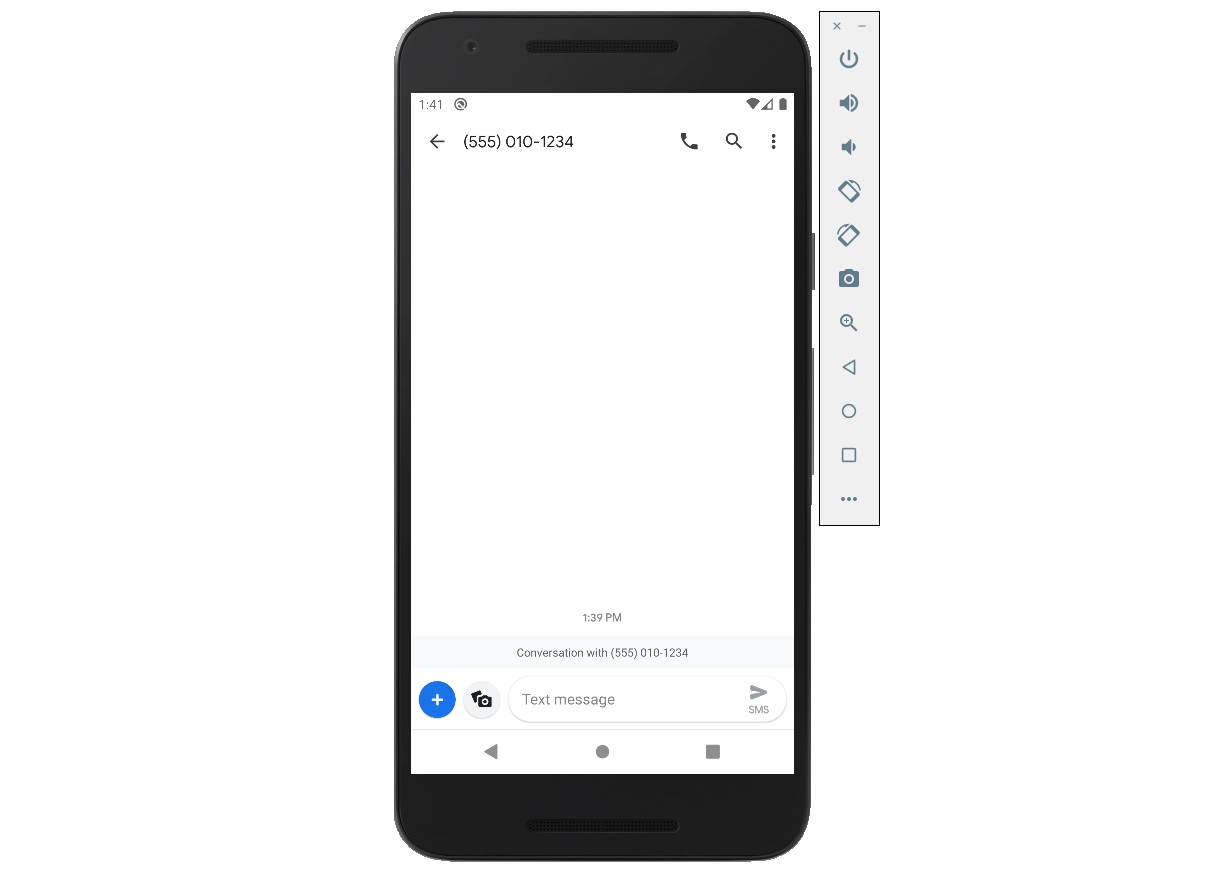
Completed
Done! we’ve seen how to use url_launcher to open the browser by URL. Also, we’ve seen how to open the other apps by URL with email, telephone number, and SMS.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.