Contents
Outline
In this blog post, I will show you how to use TTS(Text To Speech) feature in Flutter. To implement the TTS feature in Flutter, I will use the flutter_tts
package.
You can see the source code of this blog post in GitHub.
So, let’s see how to use the flutter_tts
package to implement the TTS feature in Flutter.
Create Flutter project
Execute the command below to create a new Flutter project to use the flutter_tts
package.
flutter create tts_example
Execute the command below to apply Null safety
.
cd tts_example
dart migrate --apply-changes
Install flutter_tts package
To use the TTS feature in Flutter, execute the command below to install the flutter_tts
package.
flutter pub add flutter_tts
Configure Android
We can use the flutter_tts
package in only Android SDK upper than 21. For this, open the android/app/build.gradle
file and modify it like below.
minSdkVersion 21
How to use
Le’ts see how to use the flutter_tts
package to use the TTS feature in Flutter. Open the main.dart
file and modify it like below.
import 'package:flutter/material.dart';
import 'package:flutter_tts/flutter_tts.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
debugShowCheckedModeBanner: false,
home: Home(),
);
}
}
class Home extends StatelessWidget {
final FlutterTts tts = FlutterTts();
final TextEditingController controller =
TextEditingController(text: 'Hello world');
Home() {
tts.setLanguage('en');
tts.setSpeechRate(0.4);
}
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextField(
controller: controller,
),
ElevatedButton(
onPressed: () {
tts.speak(controller.text);
},
child: Text('Speak'))
],
),
);
}
}
Let’s see the source code one by one.
import 'package:flutter_tts/flutter_tts.dart';
Import the flutter_tts
package to use it.
class Home extends StatelessWidget {
final FlutterTts tts = FlutterTts();
final TextEditingController controller =
TextEditingController(text: 'Hello world');
...
}
To use the TTS feature in Flutter, create a FlutterTts
instance, and to read the user input, create a TextEditingController
instance to prepare to get the user input.
class Home extends StatelessWidget {
...
Home() {
tts.setLanguage('en');
tts.setSpeechRate(0.4);
}
...
}
When the Home widget is created, initialize the language and configure the read speed by using the FlutterTts
feature.
class Home extends StatelessWidget {
...
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextField(
controller: controller,
),
ElevatedButton(
onPressed: () {
tts.speak(controller.text);
},
child: Text('Speak'))
],
),
);
}
...
}
Make the screen with the TextField
widget to get the user input and the ElevatedButton
widget to read the user input.
Execute
Execute the command to open the Flutter app we’ve developed above.
flutter run
Or, you can execute the app with the Editor debug feature. When the app is opened, you can see the screen like below.
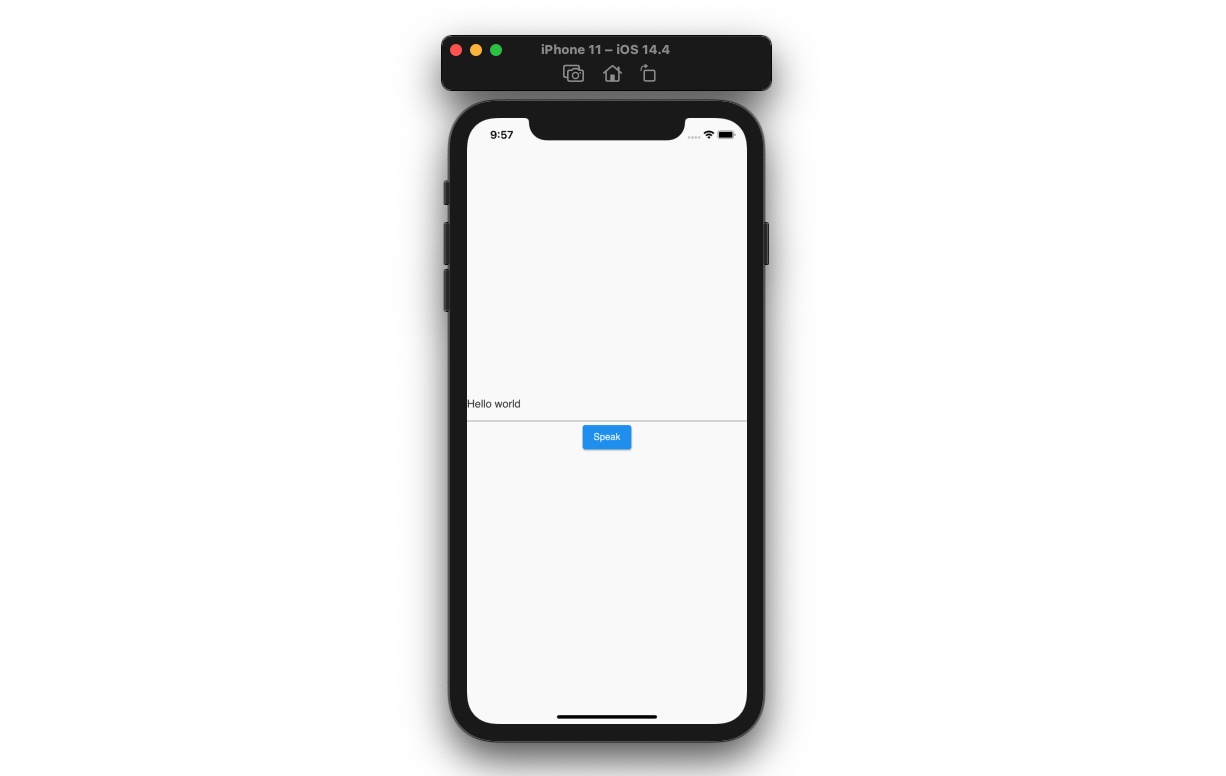
And then, when you press the Speak
button, you can hear the Hello world
voice.
When you insert other text in the TextField
widget and press the Speak
button, you can hear the text voice.
Other features
- speak: Read the text by TTS
- stop: Stop current TTS speaking.
- get languages: Get the language list provided by TTS.
- set language: Configure the TTS language
- set speech rate: Configure the TTS read speed.
- set speech volume: Configure TTS volume.
- get voices: Get the voice list provided by TTS
- set voice: Configure the TTS voice.
In addition, there are various features. See the details on the official site.
Completed
Done! we’ve seen how to configure and use the flutter_tts
package to use the TTS(Text To Speech) feature in Flutter. Now, you can provide the reading the text via the TTS feature in Flutter!
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.