Outline
In this blog post, I will introduce how to use package_info_plus
to get the app name, package name, version or build number in Flutter.
- package_info_plus: https://pub.dev/packages/package_info_plus
You can see the full source code of this blog post at the following link.
Install package_info_plus
To check how to use package_info_plus
in Flutter, execute the following command to create a new Flutter project.
flutter create package_info_plus_example
And then, execute the following command to install the package_info_plus
package.
flutter pub add package_info_plus
Next, let’s see how to use package_info_plus.
How to use
You can use package_info_plus
to get the info like the below.
import 'package:package_info_plus/package_info_plus.dart';
PackageInfo packageInfo = await PackageInfo.fromPlatform();
String appName = packageInfo.appName;
String packageName = packageInfo.packageName;
String version = packageInfo.version;
String buildNumber = packageInfo.buildNumber;
- appName: App name
- packageName: App package name
- version: App version
- buildNumber: App build number
Example
To check how to use package_info_plus
, open the lib/main.dart
file and modify it like the below.
import 'package:flutter/material.dart';
import 'package:package_info_plus/package_info_plus.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
primarySwatch: Colors.blue,
),
home: const MyHomePage(),
);
}
}
class MyHomePage extends StatelessWidget {
const MyHomePage({Key? key}) : super(key: key);
Future<PackageInfo> _getPackageInfo() {
return PackageInfo.fromPlatform();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Package Info Plus'),
),
body: Center(
child: FutureBuilder<PackageInfo>(
future: _getPackageInfo(),
builder: (BuildContext context, AsyncSnapshot<PackageInfo> snapshot) {
if (snapshot.hasError) {
return const Text('ERROR');
} else if (!snapshot.hasData) {
return const Text('Loading...');
}
final data = snapshot.data!;
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('App Name: ${data.appName}'),
Text('Package Name: ${data.packageName}'),
Text('Version: ${data.version}'),
Text('Build Number: ${data.buildNumber}'),
],
);
},
),
),
);
}
}
When the app is started, the FutureBuilder
is executed and you can get the package_info_plus
to get the information.
Future<PackageInfo> _getPackageInfo() {
return PackageInfo.fromPlatform();
}
FutureBuilder<PackageInfo>(
future: _getPackageInfo(),
builder: (BuildContext context, AsyncSnapshot<PackageInfo> snapshot) {
...
},
),
When you get the information successfully, the app name, package name, version and build number are printed.
FutureBuilder<PackageInfo>(
future: _getPackageInfo(),
builder: (BuildContext context, AsyncSnapshot<PackageInfo> snapshot) {
...
final data = snapshot.data!;
return Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text('App Name: ${data.appName}'),
Text('Package Name: ${data.packageName}'),
Text('Version: ${data.version}'),
Text('Build Number: ${data.buildNumber}'),
],
);
},
),
Check
When you execute the code, you can see the screen like the below.
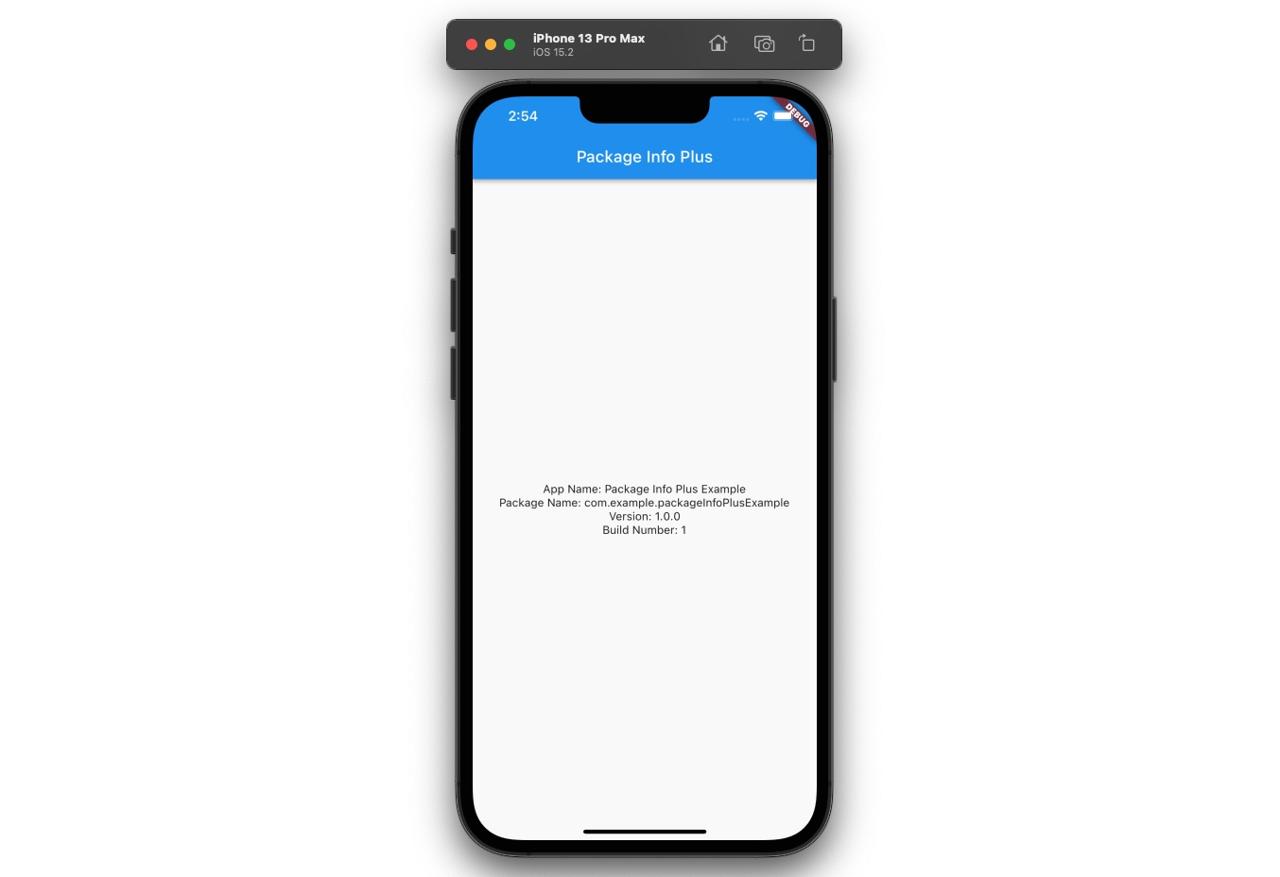
Completed
Done! we’ve seen how to use package_info_plus
to get the app name, package name, version and build number. Also, we’ve seen how to use FutureBuilder
to manage the asynchronous process.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.