Contents
Outline
Flutter provides a way to inspect and improve the accessibility of apps.
In this blog post, I will introduce how to improve the accessibility of apps developed with Flutter.
Accessibility check on Google Play Console
If you go to Google Play Console and go to Testing > Pre-launch report > Details > Accessibility
, you can see the result of the app’s accessibility check like the following.
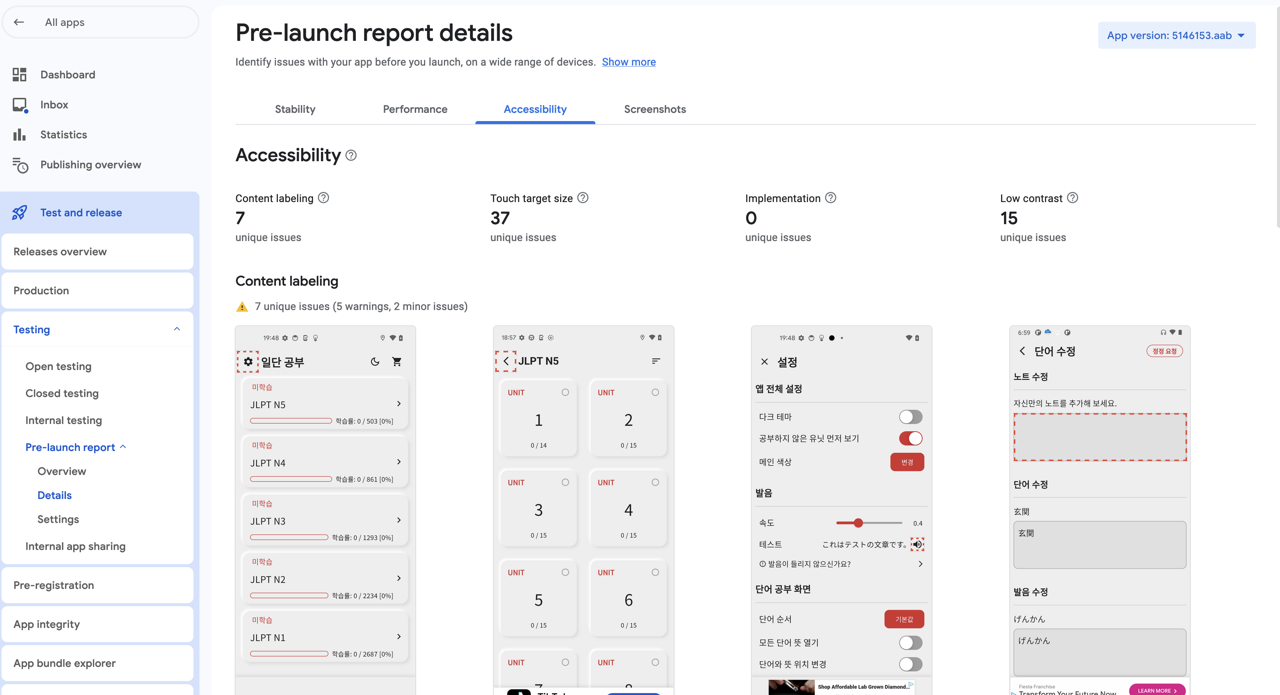
If you have conducted public/private/internal tests before deploying the app, you can see the accessibility results here. If no problems are found, the app’s accessibility can be considered good.
If problems are found here, you can improve the app’s accessibility by referring to the content introduced in this blog post.
Improving widget accessibility
Some widgets provide additional properties to improve accessibility. Even widgets without properties for improving accessibility can be improved by using the Semantics
widget.
IconButton accessibility
When using the IconButton
widget, the Screen Reader
may not recognize the widget. In this case, you can improve accessibility by wrapping the IconButton
widget with the Semantics
widget or using the Tooltip
property of the IconButton
widget.
- Example using the
Semantics
widget
Semantics(
button: true,
label: 'Add',
child: IconButton(
icon: Icon(Icons.add),
onPressed: () {},
),
)
- Example using the
Tooltip
property
IconButton(
icon: Icon(Icons.add),
onPressed: () {},
tooltip: 'Add',
)
TextField accessibility
When using the TextField
widget, you can improve accessibility by using the labelText
property or the hintText
property.
- Example using the
labelText
property
TextField(
decoration: InputDecoration(
labelText: 'Email',
),
)
- Example using the
hintText
property
TextField(
decoration: InputDecoration(
hintText: 'Email',
),
)
Using Semantics widget for accessibility improvement
You can use the Semantics
widget to improve accessibility. For example, when using the Switch
widget, you can wrap the Switch
widget with the Semantics
widget and use the label
property to improve accessibility.
Semantics(
label: 'Enable push notifications',
child: Switch(
value: _value,
onChanged: (value) {
setState(() {
_value = value;
});
},
),
)
Also, when using the InkWell
widget, you can use the Semantics
widget to improve accessibility.
Semantics(
label: 'Open profile',
child: InkWell(
onTap: () {
// Open profile
},
child: Icon(Icons.person),
),
)
Color contrast and button size
To improve accessibility, you may need to adjust the color contrast or button size. This can improve accessibility by using appropriate color contrast and button size.
To check the color contrast and size, you can use the accessibility test provided by Flutter as follows.
Accessibility test
Flutter
provides accessibility tests to check if widgets comply with accessibility guidelines. This allows you to check if widgets comply with accessibility.
Add accessibility tests to the code that tests Flutter
widgets as follows.
testWidgets('Check accessibility', (WidgetTester tester) async {
final SemanticsHandle handle = tester.ensureSemantics();
await tester.pumpWidget(TargetWidget());
await expectLater(tester, meetsGuideline(androidTapTargetGuideline));
await expectLater(tester, meetsGuideline(iOSTapTargetGuideline));
await expectLater(tester, meetsGuideline(labeledTapTargetGuideline));
await expectLater(tester, meetsGuideline(textContrastGuideline));
handle.dispose();
});
With this test code, you can check color contrast, button size, and labels recognized by screen readers.
Completed
Done! We’ve seen how to improve the accessibility of apps developed with Flutter
. We’ve also seen how to check if the app complies with accessibility guidelines using the accessibility test provided by Flutter
.
I hope you can write test code to improve the accessibility of apps developed with Flutter
and apply methods to improve accessibility.
Was my blog helpful? Please leave a comment at the bottom. it will be a great help to me!
App promotion
Deku
.Deku
created the applications with Flutter.If you have interested, please try to download them for free.